args Parameter in Java
-
args
Parameter in themain
Method in Java -
args
Parameter as an Array in themain
Method in Java -
args
Parameter asvar-args
in themain
Method in Java - Convert args Parameter to Another Data Type in Java
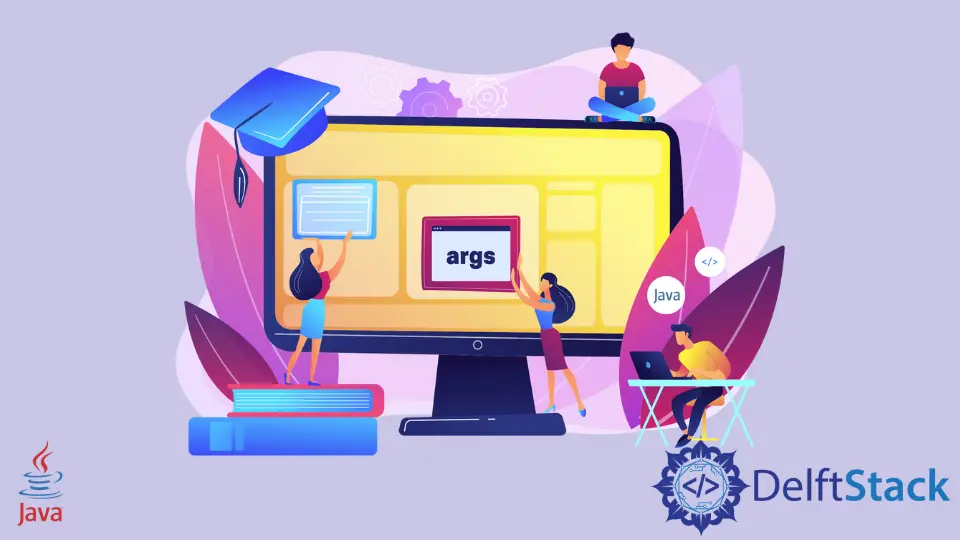
This tutorial introduces what is string args
parameter in the main method in Java.
In Java, the main
method is an entry point of execution where Java compiler starts execution. This method has a string type parameter, basically an array(args[]
). The args
name is not fixed so that we can name it anything like foo[]
, but it should be of the string type.
Java compiler uses this parameter to get command line arguments passed during the program execution. Let’s see some examples.
args
Parameter in the main
Method in Java
Here, we use a for
loop to iterate and display the command line arguments hold by the args array. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
for (int i = 0; i < args.length; i++) {
System.out.println(args[i]);
}
}
}
Below is how we can supply a command-line argument to the main method during the program execution.
Java SimpleTesting Hello DelftStack
Output:
Hello
DelftStack
args
Parameter as an Array in the main
Method in Java
Since it is an array, so we can get argument values with index bases. The first value is present at the index as 0, and the last value is present at n-1
index, where n is the array length. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String val1 = args[0];
String val2 = args[1];
System.out.println(val1);
System.out.println(val2);
}
}
}
Output:
Hello
DelftStack
args
Parameter as var-args
in the main
Method in Java
Java allows declaring the args[]
parameter as var-args which works similarly. It can also be written as the given example.
public class SimpleTesting {
public static void main(String... args) {
String val1 = args[0];
String val2 = args[1];
System.out.println(val1);
System.out.println(val2);
}
}
}
Output:
Hello
DelftStack
Convert args Parameter to Another Data Type in Java
The args[]
parameter is of the string type, so if we wish to convert its type, we can use wrapper class methods. For example, to get an integer type value, we can use the parseInt()
method. See the example below.
public class SimpleTesting {
public static void main(String... args) {
String val1 = Integer.parseInt(args[0]);
String val2 = Integer.parseInt(args[1]);
System.out.println(val1);
System.out.println(val2);
}
}
}
Run the code as below.
Java SimpleTesting 10 20
Output:
10
20