Java Aggregation vs Composition
- Composition in Java
- Aggregation in Java
- Composition vs. Aggregation
- Work with Composition in Java
- Work with Aggregation in Java
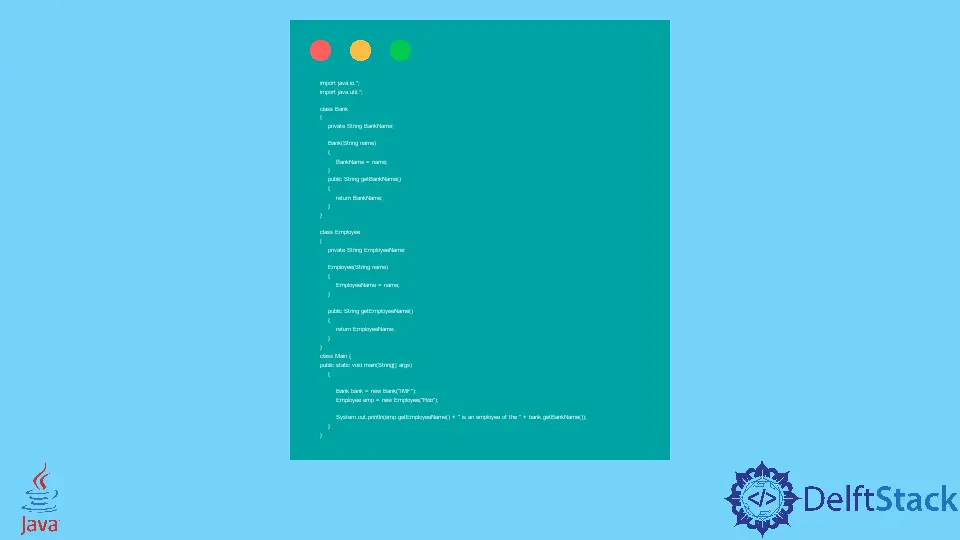
In Java, aggregation and composition are two concepts closely linked together. Composition
is a close association between classes, whereas aggregation is a weak association.
Composition in Java
Composition is when a base class object owns another derived class object, and the derived class object cannot exist without the base class.
For example, constructing a Human
will have several sub-classes: the hand, leg, and heart. When a Human
object dies, all its sub-parts cease meaningfully exist.
Aggregation in Java
Aggregation is when a base class object and its derived class object can exist independently. For example, if we construct a Player
base class and derive a Team
class from it, the team would still exist if the Player
object is destroyed.
Similarly, if the Team
is dissolved, then the Player
will still exist and can join another team.
Composition vs. Aggregation
Composition | Aggregation |
---|---|
PART-OF relation or special HAS A relation |
HAS A relation |
Unidirectional dependency | Bi-directional dependency |
Strong type of association | Weak type of association |
Child is dependent on parent class | Child is independent to parent class |
UML diagram representation is a black diamond | UML diagram representation is a white diamond |
Work with Composition in Java
import java.io.*;
import java.util.*;
class Job {
private int pay;
private int id;
public int getWage() {
return pay;
}
public void setWage(int pay) {
this.pay = pay;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
class Person {
private Job job;
public Person() {
this.job = new Job();
job.setWage(650);
}
public int getWage() {
return job.getWage();
}
}
class Main {
public static void main(String[] args) {
Person person = new Person();
int salary = person.getWage();
System.out.println("The wage of the person for a job: " + salary);
}
}
Output:
The wage of the person for a job: 650
The above code displays the relationship of composition between two classes. Composition is classified as a part-of
type of relation; in the above case, wage
is part of a job
.
We have defined parameterized constructors, setter, and getter functions for both classes.
Work with Aggregation in Java
import java.io.*;
import java.util.*;
class Bank {
private String BankName;
Bank(String name) {
BankName = name;
}
public String getBankName() {
return BankName;
}
}
class Employee {
private String EmployeeName;
Employee(String name) {
EmployeeName = name;
}
public String getEmployeeName() {
return EmployeeName;
}
}
class Main {
public static void main(String[] args) {
Bank bank = new Bank("IMF");
Employee emp = new Employee("Rob");
System.out.println(emp.getEmployeeName() + " is an employee of the " + bank.getBankName());
}
}
Output:
Rob is an employee of the IMF
The above code displays the relationship of aggregation between two classes. Aggregation is classified as a has-a
type of relation; in the above case, the bank
has an employee named Rob
.
We have defined parameterized constructors, setter, and getter functions for both classes.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn