How to Accessor Methods in Java
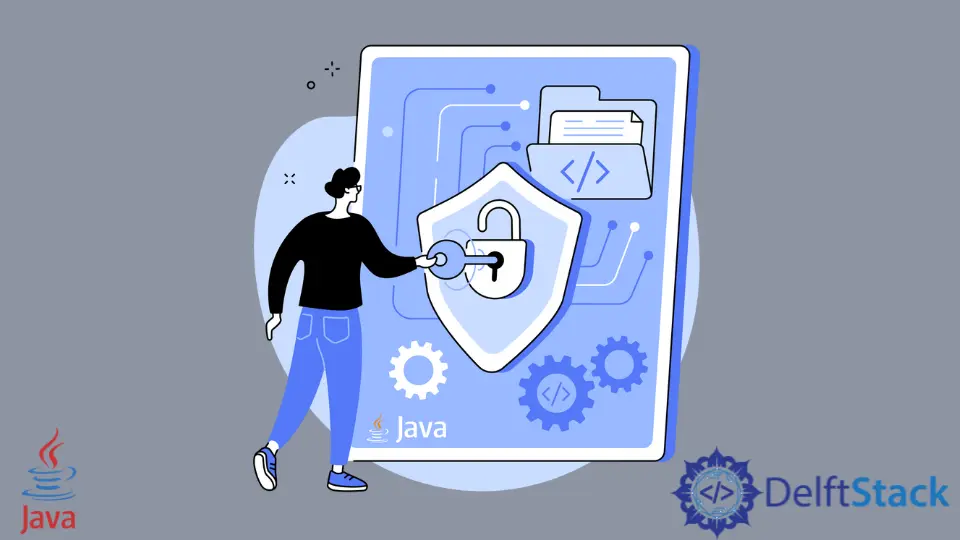
In a class, we specify the access modifiers to control the scope of different fields and methods belonging to the class. We cannot access private
and protected
members from outside the class. However, we can use the accessor methods to return the value of the private
field.
The accessor is a getter function that returns the variable or value of a class. The accessor methods are publicly declared and return the property of the object. They return the value of a private
field. The type of data returned depends on the type of the private
field.
For example,
public class student {
private int roll_number;
public int getrollNumber() // accessor usage
{
return roll_number;
}
public void setrollNumber(int newNumber) {
this.roll_number = newNumber;
}
public static void main(String[] args) {
student a = new student();
a.setrollNumber(5);
System.out.print(a.getrollNumber());
}
}
Output:
5
In the above example, the class’s getRollNumber()
function is the accessor method. It returns the value of the private
variable roll_number
.
Another essential function to notice in the above example is the function setrollNumber()
. It is a mutator method. Such functions work on a similar concept to accessors but set the value of a privately declared variable. In our example, we first set the field roll_number
value using a mutator and accessed it using an accessor.
Our major goal is to hide as much of the object’s data as possible. Therefore we need to restrict purposeful or accidental access to these items. So we use the concept of accessors and mutators, which only return the value of such variables and allow us to set their values.