The Difference Between the != and =! Operator in Java
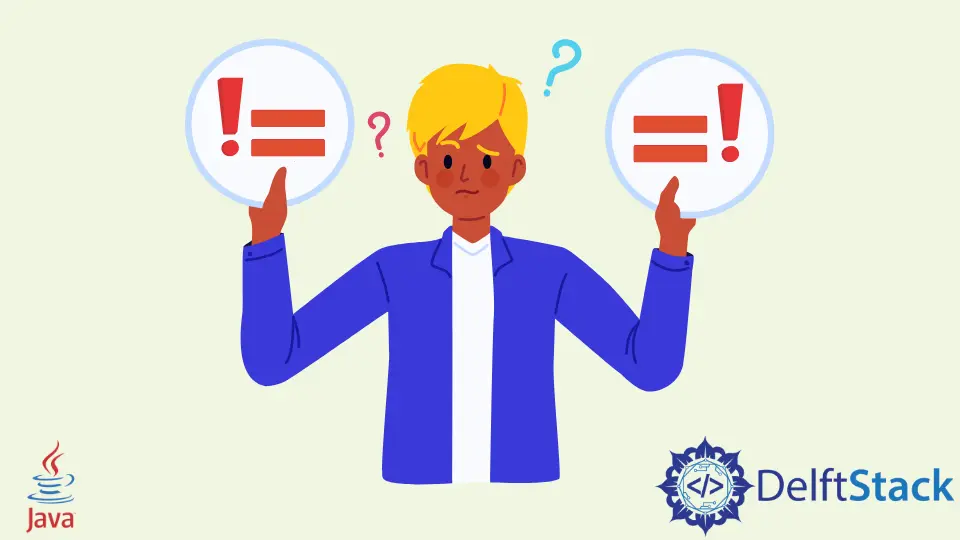
This tutorial introduces the difference between the !=
and =!
operators with examples in Java.
The !=
operator is an equality operator that is used to check whether two operands are equal or not. The =!
operator is a combination of two operators, one is an assignment, and the second is a negation operator that works on boolean value. And it is used to invert a boolean value.
There is no comparison between these as both are used for different purposes. Let’s start with some examples to understand the uses.
Java !=
Operator Example
The !=
operator, also known as not equal to
, is an equality operator and is used to check the equality of two operands. It returns a boolean value that is either true or false. If the two operands are equal, then it returns false, true otherwise.
public class SimpleTesting {
public static void main(String[] args) {
int a = 10, b = 10;
System.out.println("a = " + a);
System.out.println("b = " + b);
boolean result = a != b;
System.out.println(result);
a = 20;
System.out.println("a = " + a);
result = a != b;
System.out.println(result);
}
}
Output:
a = 10
b = 10
false
a = 20
true
The expression (a != b)
means ! (a == b)
: the opposite of a == b
can also be written as !(a==b)
. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int a = 10, b = 10;
System.out.println("a = " + a);
System.out.println("b = " + b);
boolean result = !(a == b);
System.out.println(result);
a = 20;
System.out.println("a = " + a);
result = !(a == b);
System.out.println(result);
}
}
Output:
a = 10
b = 10
false
a = 20
true
Java =!
Operator Example
The =!
operator is used to assign the opposite boolean value to a variable. The expression a=!b
is actually a= !b
. It first inverts the b
and then assigns it to a
. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
boolean a = true, b = true;
System.out.println("a = " + a);
System.out.println("b = " + b);
a = !b;
System.out.println(a);
b = false;
System.out.println("b = " + b);
a = !b;
System.out.println(a);
}
}
Output:
a = true
b = true
false
b = false
true
You can also use the =!
operator with a conditional statement to make a code conditional, as we did in the below code. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
boolean a = true, b = true;
System.out.println("a = " + a);
System.out.println("b = " + b);
if (a = !b) {
System.out.println(a); // does not execute
}
b = false;
System.out.println("b = " + b);
if (a = !b) {
System.out.println(a);
}
}
}
Output:
a = true
b = true
b = false
true