The %= Operator in Java
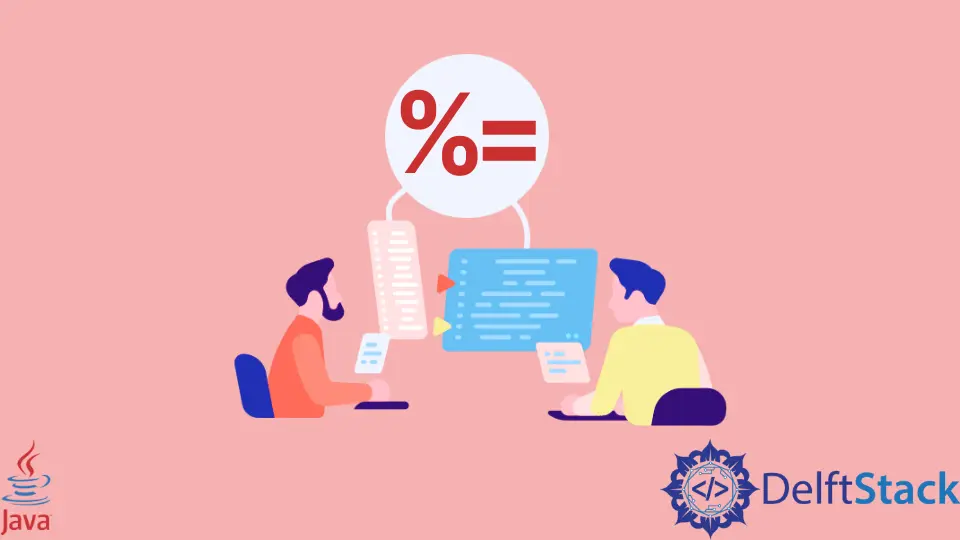
This tutorial introduces what does mean of %=
operator and how to use it in Java.
The %=
operator is a combined operator that consists of the %
(modulo) and =
(assignment) operators. This first calculates modulo and then assigns the result to the left operand.
This operator is also known as shorthand operator and is used to make code more concise. In this article, we will learn to use this operator with examples.
So, let’s start.
Modulo Operator in Java
In this example, we used the modulo operator to get the remainder of a value and then assigned it to use the assignment operator.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
int result = val % 10;
System.out.println("Remainder of " + val + "%10 = " + result);
}
}
Output:
Remainder of 125%10 = 5
Shorthand Modulo Operator in Java
Now, let’s use the shorthand operator to get the remainder. The code has concise and produces the same result as the above code did.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
int temp = val;
val %= 10; // compound operator
System.out.println("Remainder of " + temp + "%10 = " + val);
}
}
Output:
Remainder of 125%10 = 5
Shorthand Operators in Java
Java supports several other compound assignment operators such as +=, -=, *=, etc. In this example, we used other shorthand operators so that you can understand the use of these operators well.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int val = 125;
System.out.println("val = " + val);
val += 10; // addition
System.out.println("val = " + val);
val -= 10; // subtraction
System.out.println("val = " + val);
val *= 10; // multiplication
System.out.println("val = " + val);
val /= 10; // division
System.out.println("val = " + val);
val %= 10; // compound operator
System.out.println("val = " + val);
}
}
Output:
val = 125
val = 135
val = 125
val = 1250
val = 125
val = 5