How to Display Image Based on Cookies in HTML
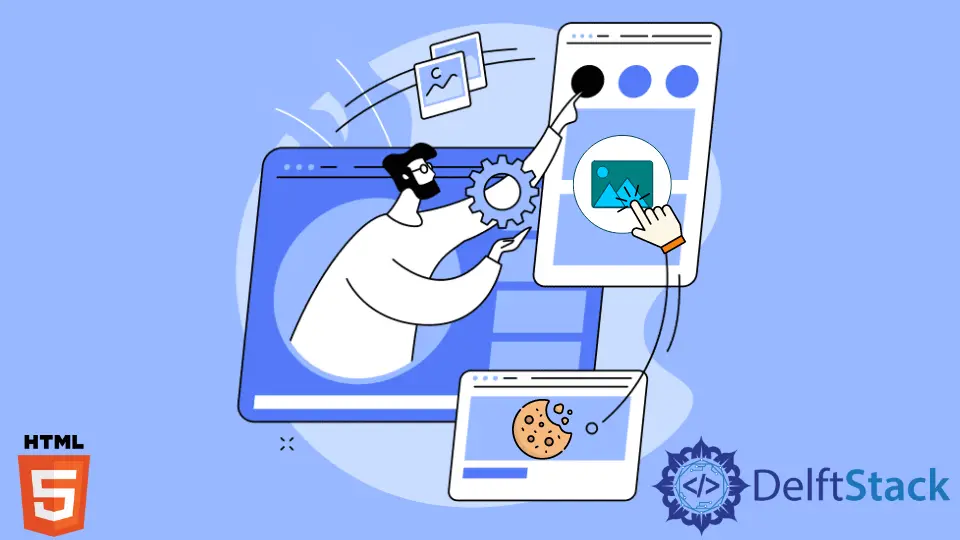
This article will introduce a way to display an image in HTML according to the cookies set in PHP.
Display Image According to Cookies Set in HTML
The problem statement is that we need to display a specific image based on the cookie passed on the webpage. We can set a cookie using the setcookie()
PHP function.
Next, the if-elseif-else
condition can be used to check the cookie. Thus, we can set the image according to the cookie and display it in HTML.
Let’s consider a scenario where a user can be directed to a webpage through 3 different web pages. We need to set the cookies differently in each of the cases.
For instance, if the user is directed through page 1, the cookie should be set to value1
. We can use the PHP rand()
function to simulate this phenomenon.
The function can be used to pick a random cookie value. For example, create an array of $values
with three items, as shown below.
$values = ["value1", "value2", "value3"];
Next, use the rand(0,2)
function as the index of the $values
array.
$value = $values[rand(0,2)];
Here, the function rand(0,2)
generates a random index between 0-2
to pick a random value from the array $values
. The random value is stored in the $value
variable.
Next, use the setcookie()
function to set a cookie named displayImage
with the value $value
. The cookie will last for an hour with time()+3600
.
Then, check the cookie name against the different values using the if-elseif-else
condition. In the if
condition, check the displayName
against $value1
.
In the if
block, create a variable $imageUrl
and set the URL abc.jpeg
. Similarly, check against $value2
and set the URL to def.jpeg
for the else if
condition.
Finally, set the URL to xyz.jpeg
in the else
condition.
In HTML, use the img
tag to insert the image. Set the src
attribute to the $imageUrl
PHP variable.
Do not forget to write the PHP variable inside the PHP tag.
Example Code:
$values = ["value1", "value2", "value3"];
$value = $values[rand(0,2)];
setcookie("displayImage", $value, time()+3600);
if($_COOKIE["displayImage"] == "value1") {
$imageUrl="parker.jpeg";
}
else if($_COOKIE["displayImage"] == "value2") {
$imageUrl="cover.jpeg";
}
else {
$imageUrl="thewanted.jpeg";
}
<img src="<?=$imageUrl?>">
The example above will randomly pick a value and set it as a cookie value. The program execution will go through the condition check, and the HTML page will display an image according to the condition.
Thus, an image can be displayed according to the cookie value set in HTML.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn