How to Create Vertical Line in HTML
-
Use the
border-left
CSS Property to Create a Vertical Line in HTML -
Tweak the
hr
Tag to Create a Vertical Line in HTML
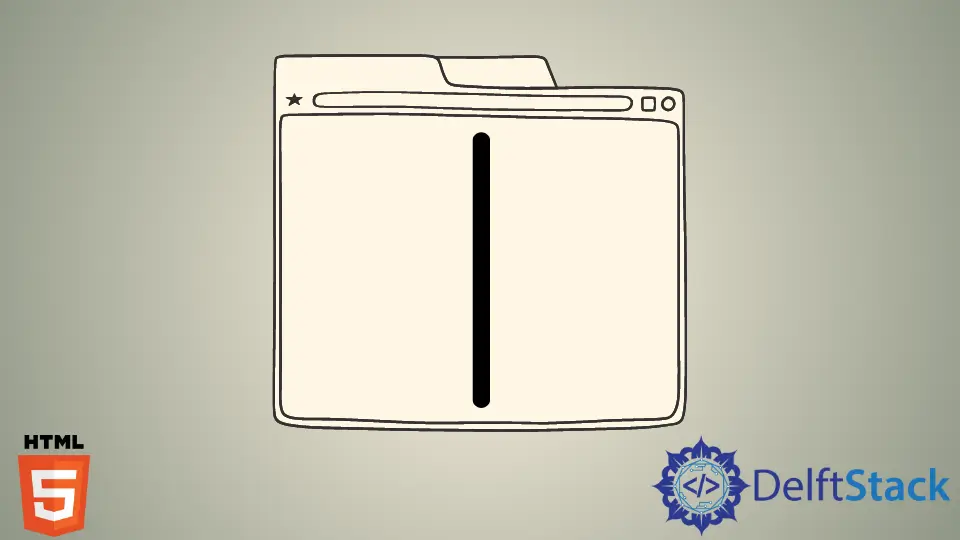
This article will introduce a few ways to create a vertical line in HTML.
Use the border-left
CSS Property to Create a Vertical Line in HTML
In HTML, we use the hr
tag to create a horizontal line, but there is no tag to create a vertical line. However, we can simulate a vertical line using the border-left
CSS property.
The border-left
property is used to style the border on the left side. The property is the shorthand of the border-left width
, border-left style
, and border-left color
.
Using only the border-left
property will create a vertical line. We can create a container in HTML, select it and apply the property to achieve a vertical line.
For example, create a div
with the class v-line
in HTML, then select v-line
and apply some styles to it. Set the border-left
property to thick solid #000
.
Next, set the height
to 100%
and left
to 50%
. Finally, set the position
property to absolute
.
Here, we will have a black border in the middle of the webpage. In this way, we can create a vertical line using the border-left
CSS property in HTML.
Example Code:
<div class="v-line">
</div>
.v-line{
border-left: thick solid #000;
height:100%;
left: 50%;
position: absolute;
}
Tweak the hr
Tag to Create a Vertical Line in HTML
We can tweak the hr
tag and create a vertical line in HTML.
The hr
tag is used to create a horizontal line. We can use the maximum value for the height
and the minimum for the width
of the hr
tag.
In this way, the height of the horizontal line will elongate, and the width will shrink. We can apply the same color for the horizontal line and its border to make it look vertical.
For example, create an hr
tag in HTML. In CSS, set the height
of the hr
tag to 100vh
and the width
to .5vw
.
Next, set the border-width
to 0
. Finally, set the color
and background-color
of the line to #000
.
Here, we set the 0
value to border-width
so that the vertical line will be thinner. In this way, we have created a vertical line using the hr
tag in HTML.
Example Code:
<hr>
hr{
height:100vh;
width:.5vw;
border-width:0;
color:#000;
background-color:#000;
}
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn