How to Transform Text Into Uppercase in HTML
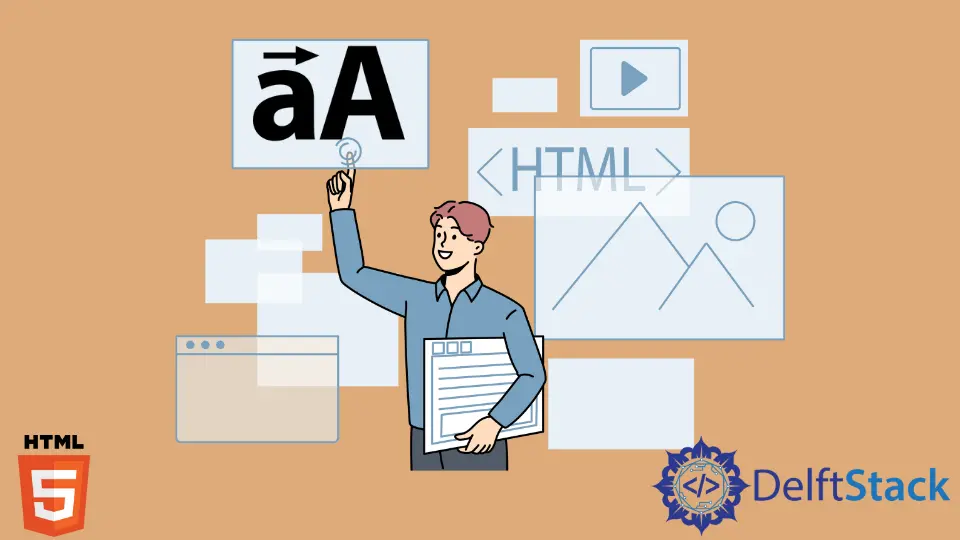
This article will introduce how to transform the text into uppercase in HTML.
Use the CSS text-transform
Property to Transform Text Into Uppercase in HTML
Capitalizing texts has never been so easier with the CSS text-transform
property. The property has several values that perform varieties of capitalization on the texts.
We can use the uppercase
value for the text-transform
property to create uppercase characters in a text. Similarly, the lowercase
value makes lowercase characters.
Using the capitalize
option, we can also capitalize only the first character of a text.
For example, create a <p>
tag and write some texts inside the paragraph. Make sure you write the text in lowercase.
In CSS, select the paragraph and use the text-transform
property. Set the value of the text-transform
property to uppercase
.
As seen in the output below, the text is transformed into uppercase. In this way, we can use the uppercase
value for the text-transform
property to write uppercase characters in HTML.
HTML Code:
<p>
Uppercase characters
</p>
CSS Code:
p {
text-transform: uppercase;
}
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn