How to Rotate Image in HTML
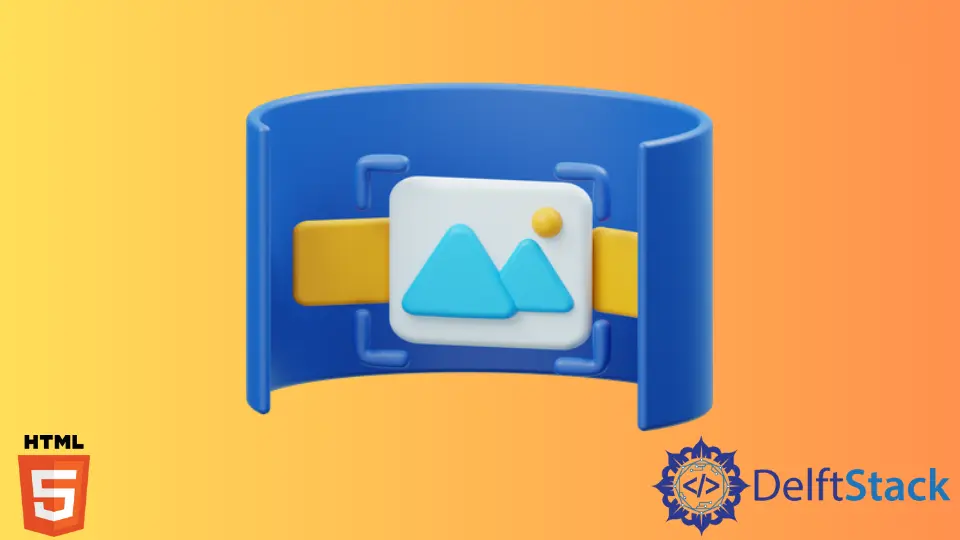
This article will introduce a method to rotate an image in HTML.
Use the transform
CSS Property to Rotate an Image in HTML
The CSS transform
property implies modifying a particular element in our code. We need an external resource, the image we transform in this case, to transform it. This property applies a 2D or 3D transformation to an element. Furthermore, this property also gives the authorization to rotate, scale, move, skew, etc., to the details. It also alters the coordinate space of the CSS visual formatting model. The elements whose layout is governed by the CSS box model can only be transformed. We can set the transform
property to a rotate()
function mentioning the unit in the function to rotate an image in HTML. The value by which the image is to be rotated should be provided in the parenthesis of the function. The deg
unit is used to specify the rotation value. We can use the inline CSS for this purpose. The CSS will be used in the <img>
tag.
For example, insert a regular image with the <img>
tag in HTML. Set the src
attribute to https://loremflickr.com/320/320
. Then, for a 90-degree rotation, insert the image and add some styles to it. In the style attribute, set the transform
property to rotate(90deg)
. Then, add a <br>
tag. Similarly, rotate the images to 180deg
and 360deg
.
The example below illustrates a method to rotate an image in an image source in HTML. We have styled the <img>
tag, meaning the image inserted can be rotated with the transform:rotate()
property. We inserted the original image and rotated the image to 90
, 180
and 360
degrees. We can write the desired degree values in the rotate()
function. The second image in the example rotates up to half of the axis. The third image looks upside down because it is rotated to 180
degrees. When the image completes a rotation of 360
degrees, it looks like the original image. Thus, we can use the transform()
property and rotate()
value to rotate the image using HTML and CSS.
Example Code:
<html>
<body>
Original Image
<img src="https://loremflickr.com/320/320" /> <br>
90 degree Rotation
<img src="https://loremflickr.com/320/320" style="transform:rotate(90deg);"> <br>
180 degree Rotation
<img src="https://loremflickr.com/320/320" style="transform:rotate(180deg);"> <br>
360 degree Rotation
<img src="https://loremflickr.com/320/320" style="transform:rotate(360deg);"> <br>
</body>
</html>
Sushant is a software engineering student and a tech enthusiast. He finds joy in writing blogs on programming and imparting his knowledge to the community.
LinkedIn