How to Redirect in HTML
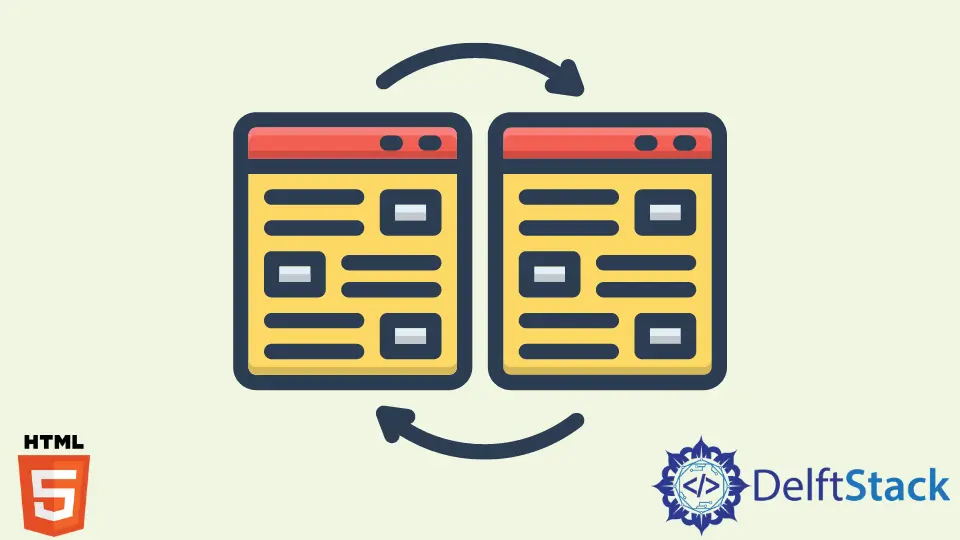
In this article, we’ll learn how to perform auto-redirect in HTML.
Use http-equiv
to Auto-Redirect a Page to Another in HTML
In HTML, we use the meta
tag to specify several metadata about the HTML document and provide many attributes to set this different information in the HTML document. The attribute called http-equiv
automatically refreshes the HTML page.
The http-equiv
attribute specifies the HTTP header for the content
attribute value. To refresh the page, we can use the content
attribute and the http-equiv
attribute in the meta
tag.
For example, create an HTML file named test.html
. Then, write the meta
tag inside the head
tag in HTML with the meta-equiv
attribute.
Set the attribute to refresh
, then write the content
attribute and give the value 1
. Without closing the quotes in the content
attribute, write the url
option and set its value to ./index.html
.
Lastly, create a file index.html
in the project’s root directory and write some text in the body
section.
Example Code:
test.html
:
<head>
<meta http-equiv="refresh" content="1; url=./index.html" />
</head>
<body>
<p> This is test.html</p>
</body>
index.html
:
<body>
this is index.html
</body>
When we open the file, test.html
, the page gets redirected to index.html
.
Sample Output:
We can also redirect the HTML page to an external website. We need to write the external website URL in the url
option in the content
attribute.
An example is shown below.
<meta http-equiv="refresh" content="1; url=https://www.upwork.com/" />
When we open the above HTML file, the page gets redirected to Upwork.
Sample Output:
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn