How to Add Hover Text in HTML
-
Use the
title
Attribute to Add a Hover Text in HTML -
Use the
title
Attribute With theabbr
Tag to Add a Hover Text in HTML
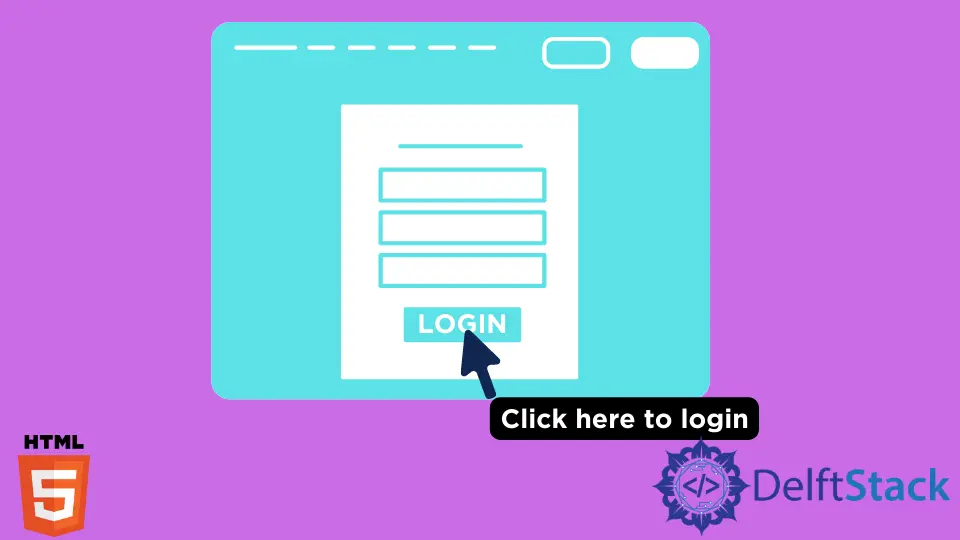
In this article, we will discuss a few ways to add a hover text in HTML. A hover text is a tooltip text that appears when we hover over a text in HTML.
Use the title
Attribute to Add a Hover Text in HTML
We can use the title
attribute to add a hover text in HTML. The attribute can be used with any HTML element.
It gives extra information about the element. When we use the title
attribute on an element, it creates a tooltip text or a hover text when we move the mouse over the element.
For example, create a Login
button with the <button>
tag. Write an attribute title
in the <button>
tag.
Next, write the text this is a login button
as the value for the title
attribute.
When we hover the mouse over the button, a hover text says this is a login button
. Here, we implemented the title
attribute with a button, an inline element.
This way, we can use the title
attribute to add a hover text in HTML.
Example Code:
<button title="this is a login button">
Login
</button>
Use the title
Attribute With the abbr
Tag to Add a Hover Text in HTML
This method discusses how we can use the title
tag to add hover text while using the abbr
tag. The title
attribute and the abbr
are usually used together.
The abbr
tag is used to write the abbreviation, and the title
tag is used to show the full form of the abbreviation during the mouse hover.
For example, consider the sentence The Undertaker is a WWE superstar
. Wrap the word WWE
with the abbr
tag.
In the tag, write the attribute title
and give it the value World Wrestling Entertainment
.
As a result, the word WWE
appears with a dotted underline. When we hover over it, a hover text will appear that says World Wrestling Entertainment
.
Thus, this is how we can use the title
attribute with the abbr
tag to create a hover text in HTML.
Example Code:
The Undertaker is a <abbr title="World Wrestling Entertainment"> WWE </abbr> superstar.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn