HTML Email Validation
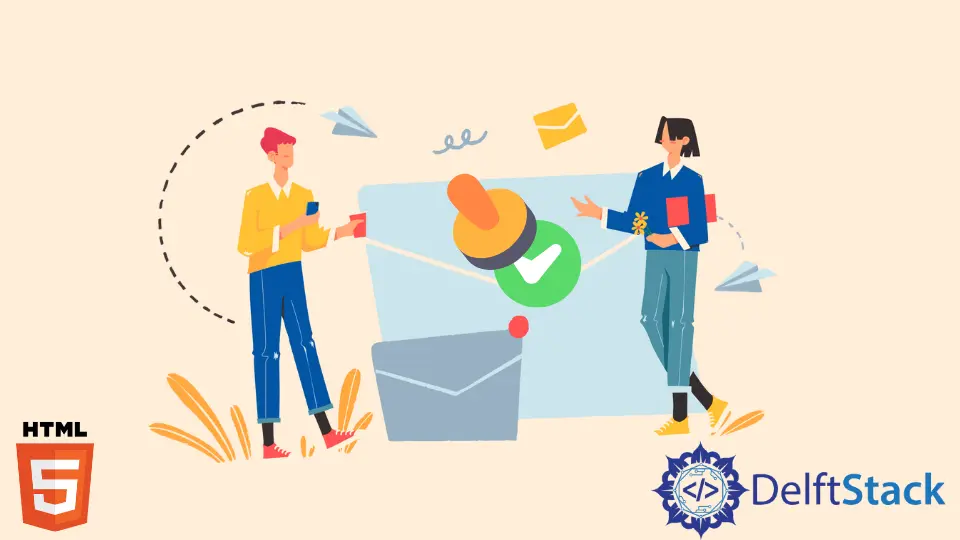
In today’s post, we’ll learn about how to do email validation in HTML.
Email Validation in HTML
The process of checking an email address to see if it is reachable and valid is known as email validation. It runs a short process that quickly finds typos, whether they are innocent mistakes or purposeful deception.
The most efficient method for ensuring the acquisition of high-quality data is still email validation. Hard bounces typically happen as a result of the email address being closed by the user, being a bogus email address, or both.
According to best practices, you should receive no more than five spam complaints for every 5,000 emails sent.
The user can enter and modify an email address using <input>
elements of type email
. Before the form can be submitted, the input value is automatically verified to ensure it is either empty or a properly formatted email address.
The value attribute of the <input>
element includes a string that is automatically validated as adhering to email syntax.
For the input value to pass constraint validation, when supplied, the pattern
attribute is a regular expression that the input’s value must match.
The pattern of the email validation is as follows:
^[a-z0-9!#$%&‘*+/=?^_`{|}~-]+(?:.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*@(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?
To further understand the previous concept, consider the following example.
<form id="emailForm">
<input
type="email"
required
placeholder="email1"
>
<input type="text" required name="email1"
pattern="^[a-z0-9!#$%&‘*+/=?^_`{|}~-]+(?:.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*@(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?">
<button type="submit">save</button>
</form>
const form = document.getElementById('emailForm');
function handleForm(event) {
event.preventDefault();
console.log('Form submitted')
}
form.addEventListener('submit', handleForm);
In the last example, we defined two input elements, the first of which is of type email
and performs email validation independently.
A valid email id pattern was supplied in the second input element of the text
data type. Incorrect email addresses will prevent the form from being submitted, and an error notice will be shown.
Run the above line of code in any browser compatible with HTML. It will display the following outcome.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn