How to Create a Download Link in HTML
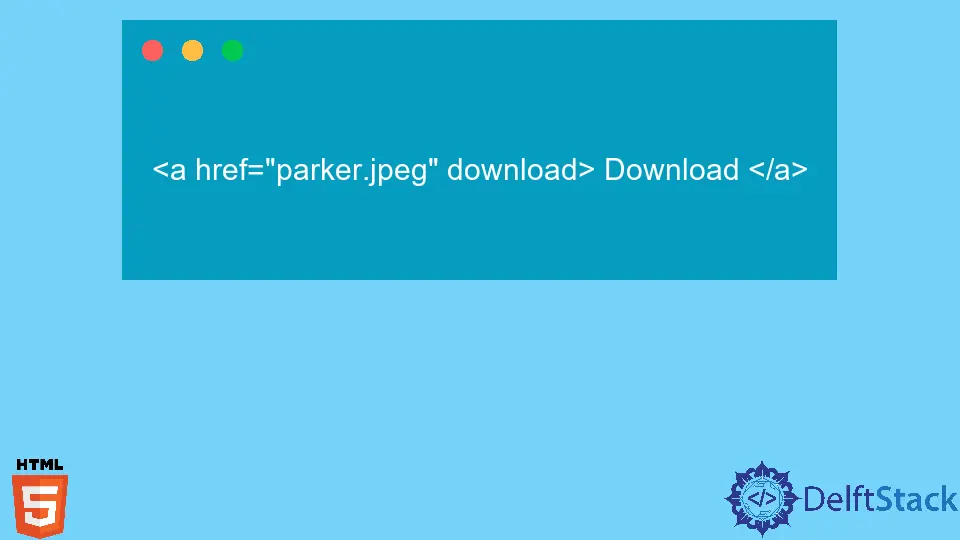
This article will introduce a way to create a download link in HTML.
Use the download
Attribute to Create a Download Link in HTML
We can use the download
attribute inside an anchor element in HTML to create a download link. In the anchor tag, we can specify the link of the file to be downloaded with the help of the href
attribute.
The anchor tag creates a hyperlink to the target file, and when we click the link, the file gets downloaded. If we set a value for the download
attribute, the file gets downloaded with that name.
For example, create a <a>
tag, and set the file’s path to be downloaded in the href
attribute. Here, the file is an image, parker.jpeg
.
Write the download
attribute next to the href
attribute. Write the text Download
between the anchor tags to create a clickable text.
Here, the image lies in the same folder as the HTML file.
Clicking on the Download
link, we will be prompted to download the file. The most important thing to consider while creating a download link is that the webpage should be hosted on a server.
We will be prompted to download the file only if the file is running on a live server. For instance, if we create an HTML file and open the file, the file will open in the browser from the local filesystem.
The URL seems something like file:///var/www/html/index.html
. In such conditions, when we click the link, the image will be displayed on the webpage, which is not what we want.
We can implement a couple of ways to run the HTML file on a server. One way is to start the local server in your system and serve the HTML file from the localhost.
It is an elegant way to run a server. Thus, we can create a download link using the download
attribute in the anchor tag in HTML.
Example Code:
<a href="/img/DelftStack/logo.png" download> Download </a>
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn