How to Center iFrame in HTML
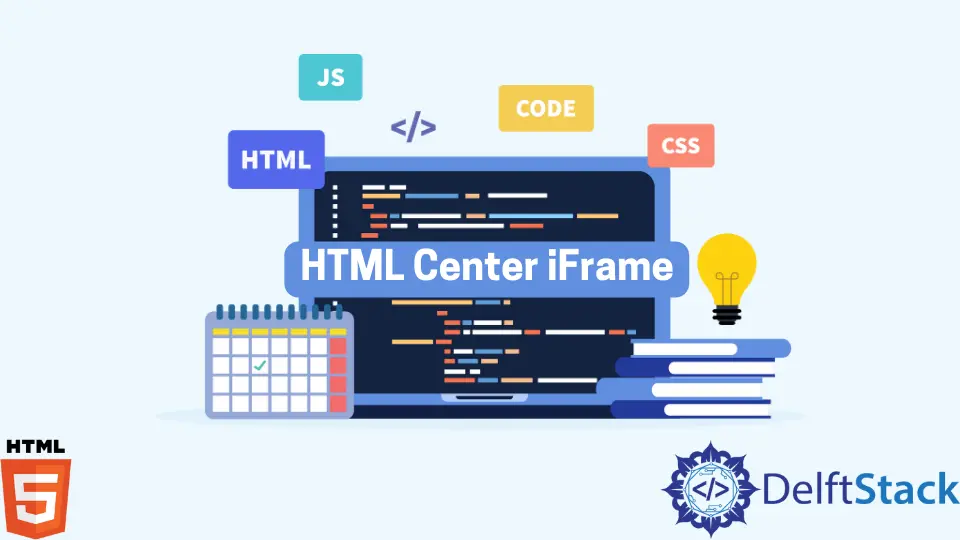
This post will look at many methods for centering an iFrame in an HTML document. The iframe
element defines an inline frame.
Use an inline frame to embed another document into the current HTML document.
Center iFrame in HTML
display : block
- An element’s rendering box type (display behavior) is specified by thedisplay
attribute.display: block
displays ablock
element like a paragraph element for an element. It fills the entire width and begins on a new line.text-align : center
- The inner content of ablock
element is aligned using thetext-align
CSS attribute. These are the conventional values fortext-align: left
, which is the default value. The left side of the content is aligned.
To further understand the previous concept, consider the following example.
<div>div</div>
<iframe class="iFrame" src="data:,iframe"></iframe>
<hr>
<div id="all">
<div>div</div>
<iframe src="data:,iframe"></iframe>
</div>
div, iframe {
width: 150px;
height: 50px;
margin: 0 auto;
background-color: #777;
}
.iFrame {
display: block;
border-style: none;
}
#all{
width: 150px;
text-align: center;
}
In the preceding example, we defined two div
with iframe
. We defined the iframe
with the display: block;
attribute in the first div
and then applied text-align: center;
to the second iframe
after enclosing it in a parent div
.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn