How to Convert HTML to JSON
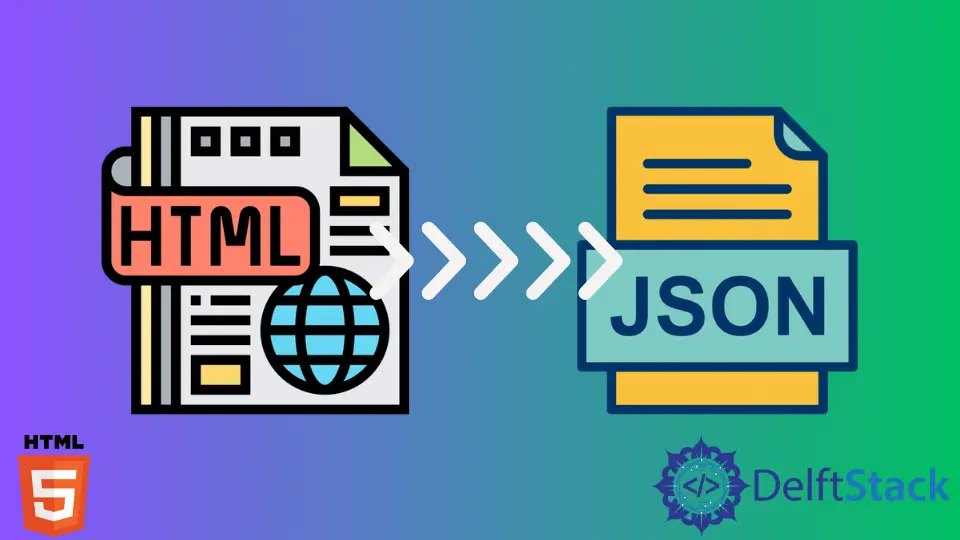
This article introduces how to convert HTML code to JSON.
Use JavaScript JSON.stringify()
Method to Convert HTML to JSON
JSON is similar to the JavaScript object, and the difference is that the key is written as a string in JSON. JSON is not language-dependent and is used to exchange data between applications or computers.
The example of a JSON string is shown below.
'{
"name":"John",
"age":30,
"car":null
}'
We can convert the HTML document in such representation using the JSON.stringify()
method. The method converts the JavaScript object into a JSON string.
For example, we will convert the following HTML document into JSON.
Code - HTML:
<div id='para'>
<p>
Paragraph 1
</p>
<p>
Paragraph 2
</p>
</div>
We need to get the document to be converted to JSON. In HTML, the id
of the container to be converted is para
.
Use the document.getElementById()
method to return the document with para
id. The para
variable stores the whole HTML document.
var para = document.getElementById('para');
Next, use the outerHTML
property to return the serialized HTML content of the para
variable. The html
variable contains the whole document in a string format.
var html = para.outerHTML;
Then, store the html
string as a JavaScript object. Name the key of the object as html
.
var obj = {html: html};
Finally, convert the object using the JSON.stringify()
method.
var json = JSON.stringify(obj);
Complete Source Code - JavaScript + Node.js:
var para = document.getElementById('para');
var html = para.outerHTML;
var obj = {html: html};
var json = JSON.stringify(obj);
console.log(json)
Output:
"{'html':'<div id=\'para\'>\n <p>\n Paragraph 1\n </p>\n <p>\n Paragraph 2\n </p>\n</div>'}"
This way, we can convert any selected HTML to JSON.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn