How to Center Multiple Links in HTML
-
Use the
<center>
HTML Tag to Center Multiple HTML Links -
Use the
text-align: center
CSS Property to Center Multiple HTML Links
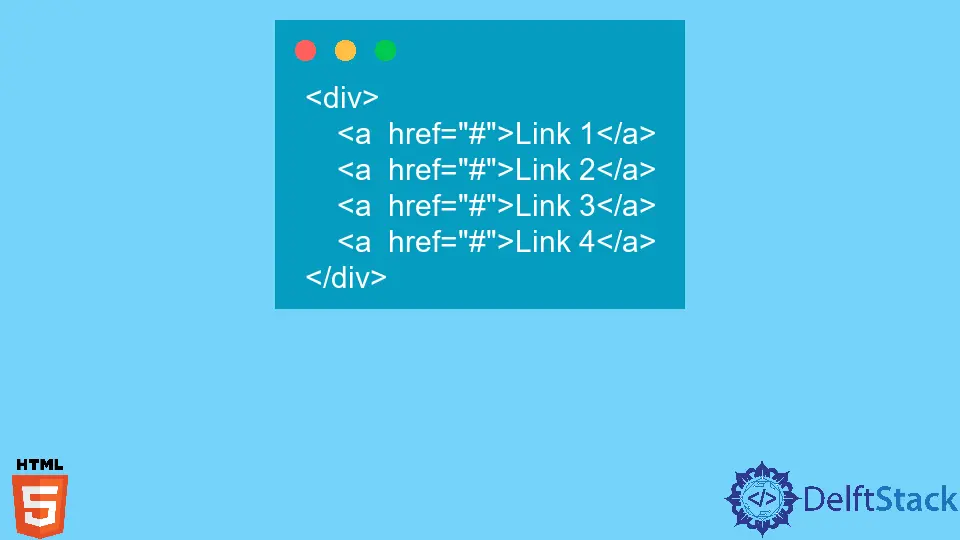
This article will teach us to center one or more HTML links. In HTML, we can use the <a>
tag to create the link and assign the URL to the src
attribute.
Use the <center>
HTML Tag to Center Multiple HTML Links
The simplest way to center an element in the HTML is the <center>
tag. We can put all the elements inside the <center> </center>
tag, which we need to display in the center.
For example, we have created four links and inserted them inside the <center>
tag to render in the center.
HTML Code:
<center>
<a href="#">About Us</a>
<a href="#">Contact Us</a>
<a href="#">HTML</a>
<a href="#">CSS</a>
</center>
In the above output, users can see that all links appear in the middle of the web page.
Use the text-align: center
CSS Property to Center Multiple HTML Links
We can also center the HTML elements using CSS’s text-align
property.
In the example below, we have created four links and added them inside the <div>
element. Next, we have applied the text-align: center
CSS property to the <div>
element, which will center all <div>
elements.
HTML Code:
<div>
<a href="#">Link 1</a>
<a href="#">Link 2</a>
<a href="#">Link 3</a>
<a href="#">Link 4</a>
</div>
CSS Code:
div{
text-align: center;
}
If users want to show all links line by line in a single column, they can add display: flex
and flex-direction: column
CSS properties for the <div>
element’s style.
HTML Code:
<div>
<a href="#">Link 1</a>
<a href="#">Link 2</a>
<a href="#">Link 3</a>
<a href="#">Link 4</a>
</div>
CSS Code:
div{
display: flex;
flex-direction: column;
text-align: center;
}
In the above output, users can observe that all links appear in a single column, and the column appears in the center of the screen.