How to Split a String With Delimiters in Go
- Using the strings Package
- Using strings.Fields for Whitespace
- Using strings.SplitN for Limited Splits
- Using Regular Expressions for Complex Delimiters
- Conclusion
- FAQ
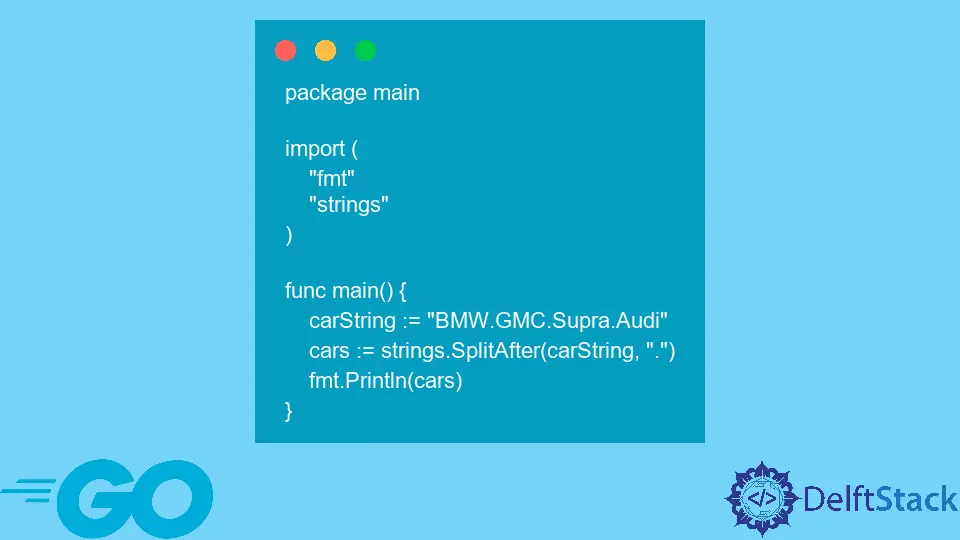
Splitting strings with delimiters is a common task in programming, and Go makes this process straightforward and efficient. Whether you’re parsing data or processing user input, knowing how to split strings can save you time and effort.
In this tutorial, we will explore different methods to split strings with delimiters in Go. We’ll cover built-in functions and libraries that can help you achieve this task with ease. By the end of this article, you’ll have a solid understanding of how to manipulate strings in Go, making your coding journey smoother and more enjoyable. So, let’s dive in!
Using the strings Package
The Go programming language comes with a powerful standard library that includes the strings
package. This package provides a handy function called Split
, which allows you to split a string based on a specified delimiter.
Here’s how you can use it:
package main
import (
"fmt"
"strings"
)
func main() {
str := "apple,banana,cherry"
fruits := strings.Split(str, ",")
fmt.Println(fruits)
}
Output:
[apple banana cherry]
In this example, we first import the necessary packages: fmt
for printing and strings
for string manipulation. We define a string str
that contains fruit names separated by commas. The strings.Split
function takes two arguments: the string to split and the delimiter (in this case, a comma). The result is a slice of strings, which we print to the console. This method is simple and effective for splitting strings based on a single delimiter.
Using strings.Fields for Whitespace
If you need to split a string based on whitespace, the strings.Fields
function is your best friend. This function splits a string into substrings separated by white space, making it ideal for parsing sentences or phrases.
Here’s how to use it:
package main
import (
"fmt"
"strings"
)
func main() {
str := "Go is an open-source programming language"
words := strings.Fields(str)
fmt.Println(words)
}
Output:
[Go is an open-source programming language]
In this code snippet, we define a string str
containing a sentence. The strings.Fields
function automatically handles multiple spaces, treating them as a single delimiter. This means you don’t have to worry about extra spaces between words. The result is a slice of words, which we then print. This method is particularly useful for natural language processing or when you want to break down sentences into individual words.
Using strings.SplitN for Limited Splits
Sometimes, you may want to limit the number of splits you perform on a string. The strings.SplitN
function allows you to specify how many substrings you want in the result. This can be particularly useful when you’re only interested in the first few elements.
Here’s an example:
package main
import (
"fmt"
"strings"
)
func main() {
str := "one:two:three:four"
parts := strings.SplitN(str, ":", 3)
fmt.Println(parts)
}
Output:
[one two three:four]
In this example, we have a string str
with multiple parts separated by colons. We use strings.SplitN
, passing in the string, the delimiter, and the maximum number of splits we want (in this case, 3). The result is a slice that contains the first two parts and the remainder of the string as the last element. This method is particularly useful when you need to preserve the remaining string after a certain number of splits.
Using Regular Expressions for Complex Delimiters
For more complex splitting scenarios, the regexp
package allows you to use regular expressions. This can be particularly useful when your delimiters are not consistent or when you need to split based on multiple criteria.
Here’s how to use it:
package main
import (
"fmt"
"regexp"
)
func main() {
str := "apple;banana orange,grape"
re := regexp.MustCompile(`[;,\s]+`)
fruits := re.Split(str, -1)
fmt.Println(fruits)
}
Output:
[apple banana orange grape]
In this example, we define a string str
that contains fruit names separated by semicolons, commas, and spaces. We compile a regular expression that matches any of these delimiters. The Split
method of the regexp
package splits the string wherever it finds a match. The result is a slice of fruit names, demonstrating how powerful regular expressions can be for splitting strings based on complex patterns.
Conclusion
In this tutorial, we’ve explored various methods to split strings with delimiters in Go. From using the built-in strings
package to leveraging regular expressions, Go provides a range of options to handle string manipulation effectively. Each method has its use cases, so understanding when to use each one is key to becoming proficient in Go programming. Whether you’re parsing data or processing user input, these techniques will enhance your coding skills and streamline your projects. Happy coding!
FAQ
-
What is the best way to split a string in Go?
The best way depends on your needs. For simple delimiters, usestrings.Split
. For whitespace, usestrings.Fields
. For limited splits, usestrings.SplitN
, and for complex patterns, use theregexp
package. -
Can I split a string by multiple delimiters in Go?
Yes, you can use theregexp
package to split a string by multiple delimiters using a regular expression that matches all desired delimiters. -
Is it possible to split a string and keep the delimiters in the result?
While the standard split functions do not keep delimiters, you can achieve this using regular expressions by capturing the delimiters in the split pattern. -
How do I handle errors when splitting strings in Go?
The string splitting functions in Go do not return errors, as they are designed to handle cases gracefully. However, you should ensure your input is valid to avoid unexpected results. -
Can I split a string in Go without using any packages?
While you can manually iterate through a string and split it, using the built-instrings
orregexp
packages is much more efficient and cleaner.