How to Reverse an Array in Go
- Method 1: Using a Simple Loop
-
Method 2: Using the Go Built-in
copy
Function - Method 3: Using Recursion
- Conclusion
- FAQ
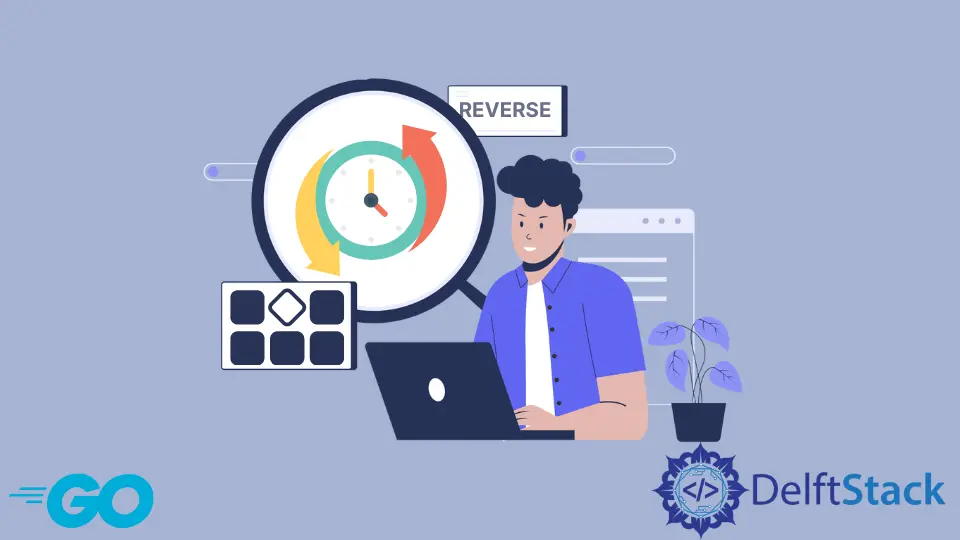
Reversing an array is a common task in programming, and Go, with its simplicity and efficiency, makes it easy to achieve. Whether you’re a beginner or an experienced developer, knowing how to manipulate arrays is essential.
In this tutorial, we will explore various methods to reverse an array in Go. We will cover different approaches, including using loops and built-in functions. By the end of this article, you will have a solid understanding of how to reverse an array in Go, complete with practical examples and clear explanations. So, let’s dive in!
Method 1: Using a Simple Loop
One of the most straightforward ways to reverse an array in Go is by using a simple loop. This method involves iterating through the array and swapping elements from the start and end until you reach the middle. Here’s how you can do it:
package main
import "fmt"
func reverseArray(arr []int) []int {
for i, j := 0, len(arr)-1; i < j; i, j = i+1, j-1 {
arr[i], arr[j] = arr[j], arr[i]
}
return arr
}
func main() {
arr := []int{1, 2, 3, 4, 5}
reversed := reverseArray(arr)
fmt.Println(reversed)
}
Output:
[5 4 3 2 1]
In this code, we define a function reverseArray
that takes an array of integers as input. It uses two indices, i
and j
, to traverse the array from both ends. The elements at these indices are swapped until they meet in the middle. This method is efficient and works in O(n) time complexity, making it suitable for larger arrays.
Method 2: Using the Go Built-in copy
Function
Another effective way to reverse an array in Go is by utilizing the built-in copy
function. This method involves creating a new array and copying elements in reverse order. Here’s how it works:
package main
import "fmt"
func reverseArray(arr []int) []int {
reversed := make([]int, len(arr))
for i := range arr {
reversed[len(arr)-1-i] = arr[i]
}
return reversed
}
func main() {
arr := []int{1, 2, 3, 4, 5}
reversed := reverseArray(arr)
fmt.Println(reversed)
}
Output:
[5 4 3 2 1]
In this example, we create a new slice called reversed
with the same length as the original array. We then loop through the original array and assign elements to the new array in reverse order. This method is also efficient, but it requires additional memory for the new array. It’s a great option when you want to keep the original array unchanged.
Method 3: Using Recursion
If you’re looking for a more advanced technique, you can reverse an array using recursion. This method may not be the most efficient, but it’s an interesting way to approach the problem. Here’s the code:
package main
import "fmt"
func reverseArray(arr []int) []int {
if len(arr) == 0 {
return arr
}
return append([]int{arr[len(arr)-1]}, reverseArray(arr[:len(arr)-1])...)
}
func main() {
arr := []int{1, 2, 3, 4, 5}
reversed := reverseArray(arr)
fmt.Println(reversed)
}
Output:
[5 4 3 2 1]
In this code, the reverseArray
function checks if the array is empty. If not, it takes the last element and appends it to the result of a recursive call on the rest of the array. While this method is elegant and showcases the power of recursion, it comes with a higher time complexity and may not be suitable for very large arrays due to stack overflow risks.
Conclusion
Reversing an array in Go can be accomplished in several ways, each with its pros and cons. Whether you choose to use a simple loop, the built-in copy
function, or recursion, understanding these methods will enhance your programming skills. As you practice, you’ll find that each approach has its place depending on the specific requirements of your project. So, experiment with these techniques and see which one works best for you!
FAQ
-
How do I reverse a string in Go?
You can convert the string to a slice of runes, reverse it using any of the methods discussed, and convert it back to a string. -
What is the time complexity of reversing an array in Go?
The time complexity is O(n) for most methods, as you need to traverse the entire array. -
Can I reverse a slice instead of an array in Go?
Yes, the same methods can be applied to slices in Go since slices are more flexible and commonly used. -
Is there a built-in function to reverse an array in Go?
No, Go does not have a built-in function specifically for reversing arrays, but you can implement it easily using the methods discussed. -
What are the common use cases for reversing an array?
Reversing an array is useful in algorithms, data manipulation, and when you need to display data in a different order.