How to Optional Parameters in Go
Jay Singh
Feb 02, 2024
Go
Go Variadic
-
Use the
variadic
Function to Pass Optional Parameters in Go -
Use the
variadic
Function to Pass Multiple Strings in Go
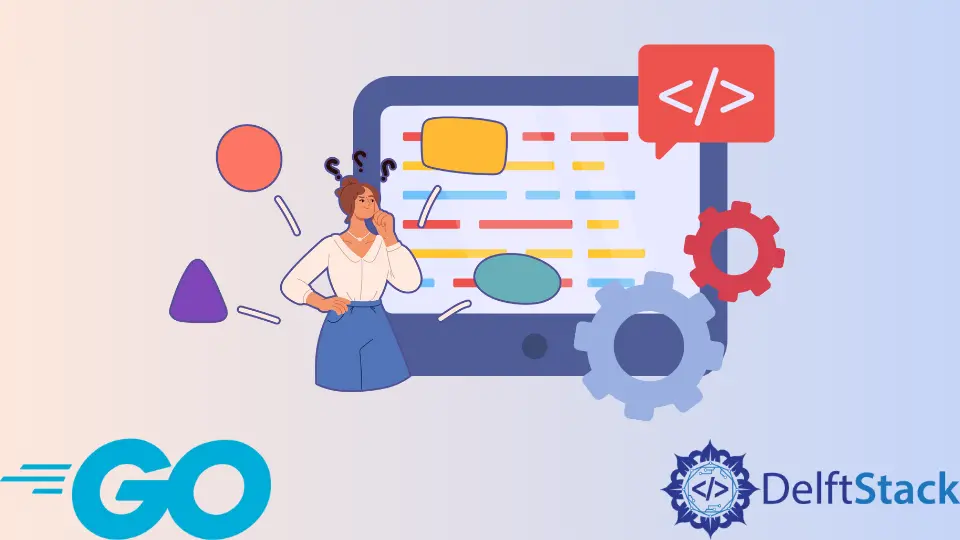
The variadic
functions have a variable number of arguments when invoked. It can be used with zero or more arguments.
An ellipsis ...
can define a variable function before a parameter.
Use the variadic
Function to Pass Optional Parameters in Go
The function will accept any number of ints
as inputs. If you already have many args
in a slice, use func(slice...)
to apply them to a variadic
function.
package main
import "fmt"
func sum(nums ...int) {
fmt.Print(nums, " ")
total := 0
for _, num := range nums {
total += num
}
fmt.Println(total)
}
func main() {
sum(1, 2)
sum(1, 2, 3)
nums := []int{1, 2, 3, 4}
sum(nums...)
}
Output:
[1 2] 3
[1 2 3] 6
[1 2 3 4] 10
Use the variadic
Function to Pass Multiple Strings in Go
The number of parameters supplied to the s
parameter has no restriction. The tree-dotted ellipsis informs the compiler that this string can have any number of values from zero to many.
package main
import "fmt"
func main() {
variadic()
variadic("white", "black")
variadic("white", "black", "blue")
variadic("white", "black", "blue", "purple")
}
func variadic(s ...string) {
fmt.Println(s)
}
Output:
[]
[white black]
[white black blue]
[white black blue purple]
Let’s make a program that says hello to folks when their names are supplied to the function:
package main
import "fmt"
func main() {
sayHello()
sayHello("Jay")
sayHello("Jessica", "Mark", "Jonas")
}
func sayHello(names ...string) {
for _, n := range names {
fmt.Printf("Hello %s\n", n)
}
}
Output:
Hello Jay
Hello Jessica
Hello Mark
Hello Jonas
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe