Lambda Expression in Golang
Jay Singh
Apr 26, 2022
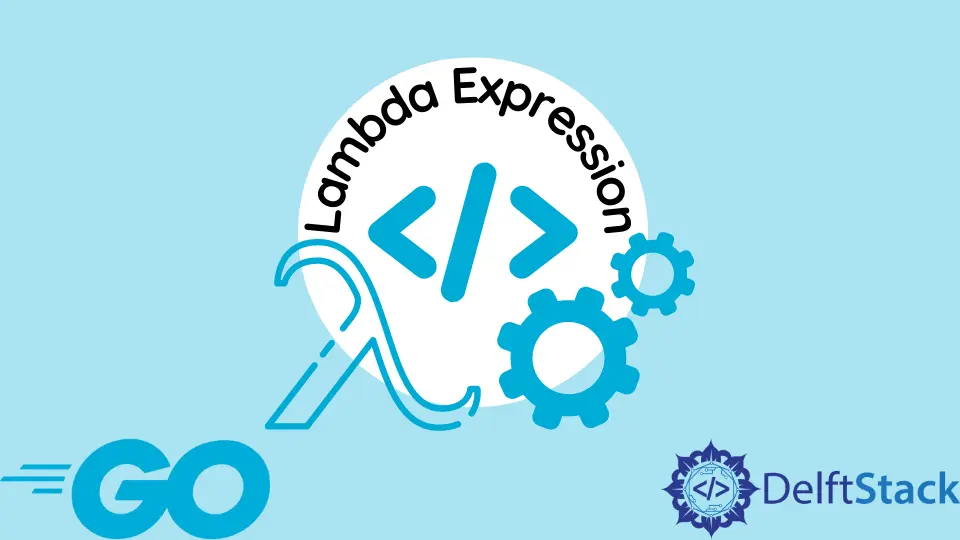
Lambda expressions do not appear to exist in Golang. A function literal, lambda function, or closure is another name for an anonymous function.
The mathematical evaluation of an expression in the lambda calculus gave rise to the idea of closure. There is a technical distinction between an anonymous function and a closure: an anonymous function is a function that has no name, whereas a closure is a function instance.
We’ll look at how to make a lambda expression in Go in this article.
Return Value From Anonymous Function in Go
In this example, the sum variable has been given the anonymous function func(n1,n2 int) int
. The sum of n1
and n2
is calculated and returned by the function.
Example:
package main
import "fmt"
func main() {
var sum = func(n1, n2 int) int {
sum := n1 + n2
return sum
}
result := sum(5, 3)
fmt.Println("Sum is:", result)
}
Output:
Sum is: 8
Return Area Using Anonymous Function in Go
In this example, the anonymous function returns the area of l*b
.
package main
import "fmt"
var (
area = func(l int, b int) int {
return l * b
}
)
func main() {
fmt.Println(area(10, 10))
}
Output:
100