How to Import Local Packages in Go
- The Package Directory in Go
- The Main Package in Go
-
Use the
go mod init
to Initialize Module in Go - Place The Source Code Files to Create Package in Go
-
Use the
import()
Method to Import Local Packages in Go
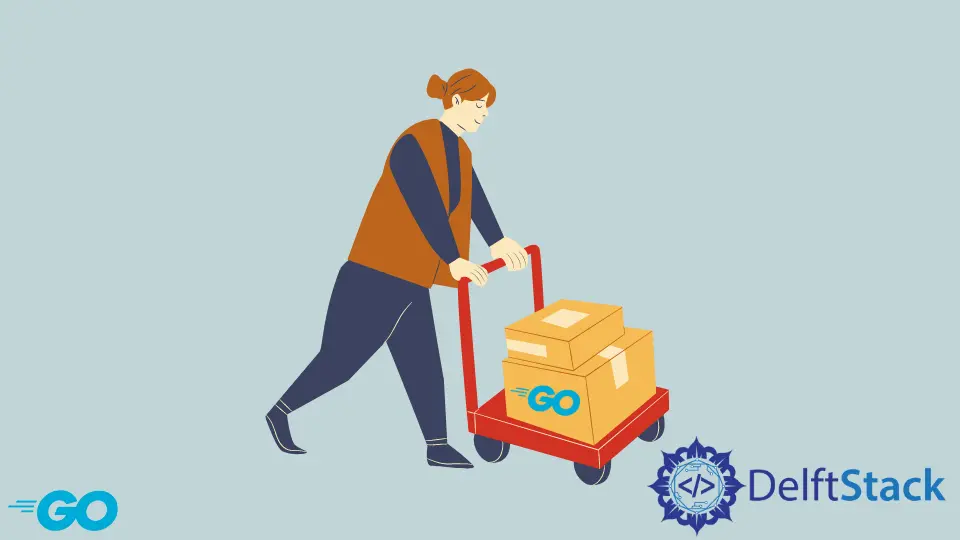
A package in Go is a collection of compiled Go source code files in the same directory.
Typically, these packages will export and import code between them. As a result, the code is modular and easy to maintain.
The Package Directory in Go
There are two packages in the workspace project: dir1
and dir2
. Each file produced directory is included in the package directory.
└───workspace
├───dir1
├───dir2
The Main Package in Go
A package’s code can be exported from any file. Then, other project files can refer to that package directory and import the code.
This allows you to import a single package and access all of the code included in the files contained within that package.
// for files in dir1
package dir1
// for files in dir2
package dir2
Use the go mod init
to Initialize Module in Go
When importing packages, the first step is to create a new module.
Syntax:
go mod init <module_name>
For instance, under the workspace directory, we can build a new module as follows:
go mod init workspace
The file provides the module name and the Go version because we don’t have any external packages.
module workspace
go 1.17
Place The Source Code Files to Create Package in Go
Build a new directory in your project and place the source code files beneath it to create a package.
$ touch dir1/dir1.go
$ touch dir2/dir2.go
Let’s add some code to each of these files now.
// dir1.go
package dir1
funcHello1() string {
return "Hello everyone, I am from dir 1"
}
//dir2.go
package dir2
funcHello2() string {
return "Hello everyone, I am from dir 2"
}
Use the import()
Method to Import Local Packages in Go
The final step is to import your local packages to use the code in each one.
Create a main.go
file at the root of your project, also known as the workspace directory.
package main
import (
"fmt"
"workspace/dir1"
"workspace/dir2"
)
funcmain() {
fmt.Println(dir1.Hello1())
fmt.Println(dir2.Hello2())
}
Output:
Hello everyone, I am from dir 1
Hello everyone, I am from dir 2