The Idiomatic Go Equivalent of C's Ternary Operator
Jay Singh
Oct 12, 2023
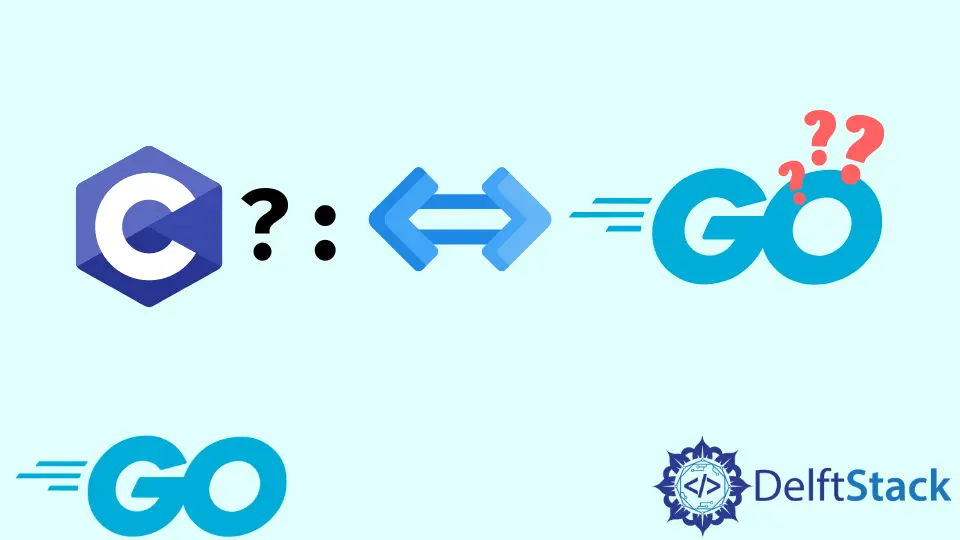
A ternary operator is a three-operand operator that aids programmers in making decisions. It’s a condensed version of the if-else
conditional.
The ternary operator is named because it requires three operators to complete. There is a ternary
operator (?:
) that evaluates like an if-else
chain in most programming languages, but there is no ternary
operator in Go.
Implementing C’s Ternary Operator in Golang
An example of a ternary
operator in the C programming language is given below, and then we will convert it into Golang.
#include <stdio.h>
int main() {
int x = 5, y = 10, result;
result = (x > y) ? x : y;
printf("%d", result);
}
Output:
10
So the ternary
operator is not available in Go. You can use an if-else
block instead, like the following examples below.
Example 1:
package main
import (
"fmt"
)
func main() {
var x, y, result int
x = 5
y = 10
if x > y {
result = x
} else {
result = y
}
fmt.Println(result)
}
Output:
10
Example 2:
v = f > 0 ? 1 : 0 // if f > 0 then v is 1 else v is 0
The solution is an if-else
block. It depicts the same code in a much more readable manner.
package main
import (
"fmt"
)
func main() {
var f, result int
f = 5
if f > 0 {
result = 1
} else {
result = 0
}
fmt.Println(result)
}
Output:
1