How to Write Multiline Strings in Go
- Understanding Multiline Strings in Go
- Creating a Simple Multiline String
- Using Multiline Strings for SQL Queries
- Handling Special Characters in Multiline Strings
- Conclusion
- FAQ
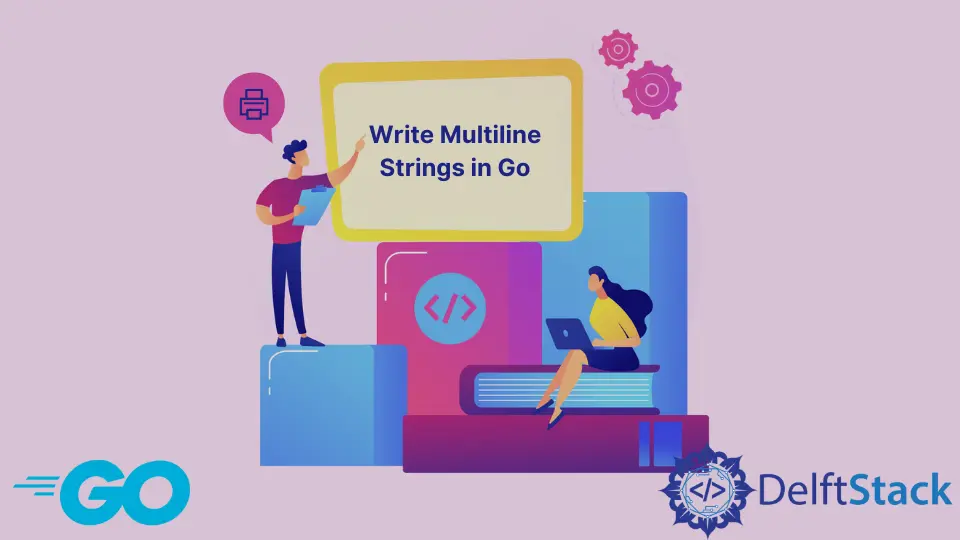
When it comes to programming in Go, handling strings efficiently is crucial. One of the most common tasks is writing multiline strings, which can be a bit tricky if you’re not familiar with the syntax. In Go, we utilize backquotes to create multiline strings, allowing us to include line breaks and tab characters without any hassle. This feature makes it easy to format text, such as generating documentation or writing complex queries.
In this article, we will explore how to write multiline strings in Go and provide examples that showcase their practical applications. Whether you’re a beginner or looking to brush up on your Go skills, this guide will help you understand the nuances of multiline strings.
Understanding Multiline Strings in Go
In Go, a multiline string can be created using backquotes (`
). This method allows you to write strings that span multiple lines without the need for concatenation or escape sequences. The content within the backquotes is treated literally, meaning that special characters like newlines and tabs are preserved as they are. This is particularly useful when you want to maintain the formatting of the text, such as when writing SQL queries or HTML templates.
Using backquotes also means you don’t have to worry about escaping characters like double quotes or backslashes, which can simplify your code significantly. Let’s dive into some examples to see how this works in practice.
Creating a Simple Multiline String
Here’s a basic example of creating a multiline string in Go:
package main
import "fmt"
func main() {
multilineString := `This is a multiline string.
It spans multiple lines.
This line is indented.`
fmt.Println(multilineString)
}
Output:
This is a multiline string.
It spans multiple lines.
This line is indented.
In this example, we define a variable called multilineString
that holds a string spanning three lines. When we print it using fmt.Println
, the output retains the original formatting, including the indentation on the last line. This demonstrates how backquotes allow for clean and readable multiline strings.
Using Multiline Strings for SQL Queries
One of the most common applications for multiline strings in Go is in database queries. When writing SQL commands, maintaining readability is essential. Here’s how you can format a SQL query using a multiline string:
package main
import "fmt"
func main() {
sqlQuery := `SELECT id, name, age
FROM users
WHERE age > 18
ORDER BY age DESC;`
fmt.Println(sqlQuery)
}
Output:
SELECT id, name, age
FROM users
WHERE age > 18
ORDER BY age DESC;
In this example, the SQL query is formatted as a multiline string, making it easy to read and maintain. The use of backquotes allows you to include line breaks without any additional syntax, which is particularly useful for complex queries with multiple clauses. This approach is not only cleaner but also reduces the chances of syntax errors.
Handling Special Characters in Multiline Strings
Another benefit of using multiline strings in Go is the ability to include special characters without escaping them. For instance, if you want to include quotes or backslashes, you can do so directly. Here’s an example:
package main
import "fmt"
func main() {
specialString := `He said, "Hello, World!"
This is a backslash: \`
fmt.Println(specialString)
}
Output:
He said, "Hello, World!"
This is a backslash: \
In this case, the string includes double quotes and a backslash without any escape sequences. This makes the code cleaner and easier to understand. By using backquotes, you can focus more on the content of your strings rather than worrying about escaping characters.
Conclusion
In summary, writing multiline strings in Go is straightforward and efficient, thanks to the use of backquotes. This feature allows you to create strings that maintain their formatting, making your code cleaner and more readable. Whether you’re working with SQL queries, HTML templates, or simply need to format text, multiline strings can significantly enhance your coding experience in Go. By understanding how to leverage this functionality, you can write more effective and maintainable code.
FAQ
-
What is a multiline string in Go?
A multiline string in Go is a string that spans multiple lines, created using backquotes. -
Can I include special characters in multiline strings?
Yes, special characters like quotes and backslashes can be included without escaping in multiline strings. -
How do multiline strings improve code readability?
They allow for better formatting and organization of text, making complex strings easier to read and maintain. -
Are there any limitations to using multiline strings in Go?
Multiline strings cannot include backquotes within them unless they are escaped. -
Can I use multiline strings for JSON data?
Yes, you can use multiline strings to format JSON data, but ensure the syntax remains valid.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn