How to Find a Type of an Object in Go
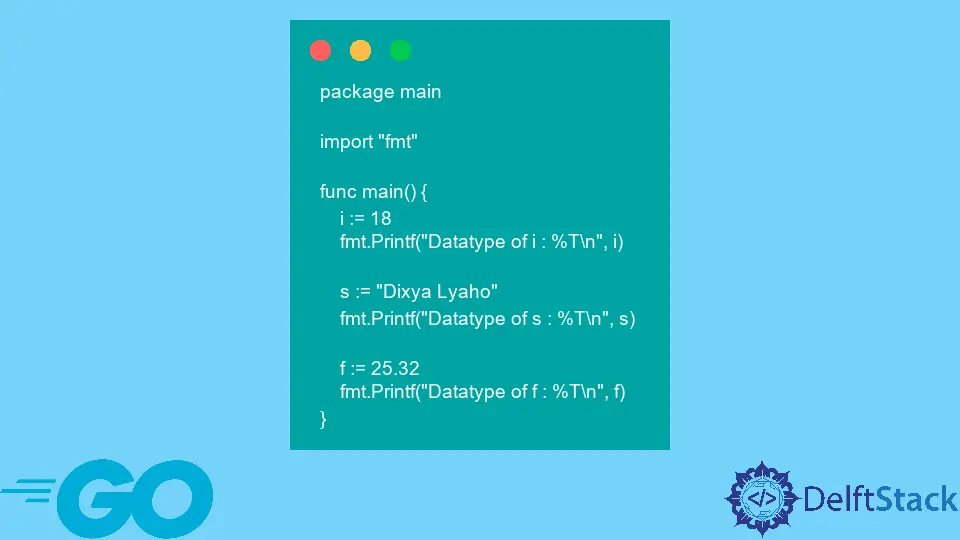
Data types specify the type associated with a valid Go variable. There are four categories of datatypes in Go which are as follows:
-
Basic type: Numbers, strings, and booleans
-
Aggregate type: Array and structs
-
Reference type: Pointers, slices, maps, functions, and channels
-
Interface type
We can find the data type of an object in Go using string formatting
, reflect
package, and type assertions
.
String Formatting to Find Data Type in Go
We can use the Printf
function of package fmt
with a special formatting option. The available formatting options to display variables using fmt
are:
Format | Description |
---|---|
%v |
Print the variable value in a default format |
%+v |
Add field names with the value |
%#v |
a Go-syntax representation of the value |
%T |
a Go-syntax representation of the type of the value |
%% |
a literal percent sign; consumes no value |
We use the %T
flag in the fmt
package to find a type of an object in Go.
package main
import "fmt"
func main() {
i := 18
fmt.Printf("Datatype of i : %T\n", i)
s := "Dixya Lyaho"
fmt.Printf("Datatype of s : %T\n", s)
f := 25.32
fmt.Printf("Datatype of f : %T\n", f)
}
Output:
Datatype of i : int
Datatype of s : string
Datatype of f : float64
reflect
Package
We can also use the reflect
package to find datatype of an object. The Typeof
function of reflect
package returns a data type that could be converted to a string
with .String()
.
package main
import (
"fmt"
"reflect"
)
func main() {
o1 := "string"
o2 := 10
o3 := 1.2
o4 := true
o5 := []string{"foo", "bar", "baz"}
o6 := map[string]int{"apple": 23, "tomato": 13}
fmt.Println(reflect.TypeOf(o1).String())
fmt.Println(reflect.TypeOf(o2).String())
fmt.Println(reflect.TypeOf(o3).String())
fmt.Println(reflect.TypeOf(o4).String())
fmt.Println(reflect.TypeOf(o5).String())
fmt.Println(reflect.TypeOf(o6).String())
}
Output:
string
int
float64
bool
[]string
map[string]int
type assertions
Method
Type assertion method returns a boolean variable to tell whether assertion operation succeeds or not. We use a type switch
to do several type assertions in series to find the data type of the object.
package main
import (
"fmt"
)
func main() {
var x interface{} = 3.85
switch x.(type) {
case int:
fmt.Println("x is of type int")
case float64:
fmt.Println("x is of type float64")
default:
fmt.Println("x is niether int nor float")
}
}
Output:
x is of type float64
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn