How to Check if a Map Contains a Key in Go
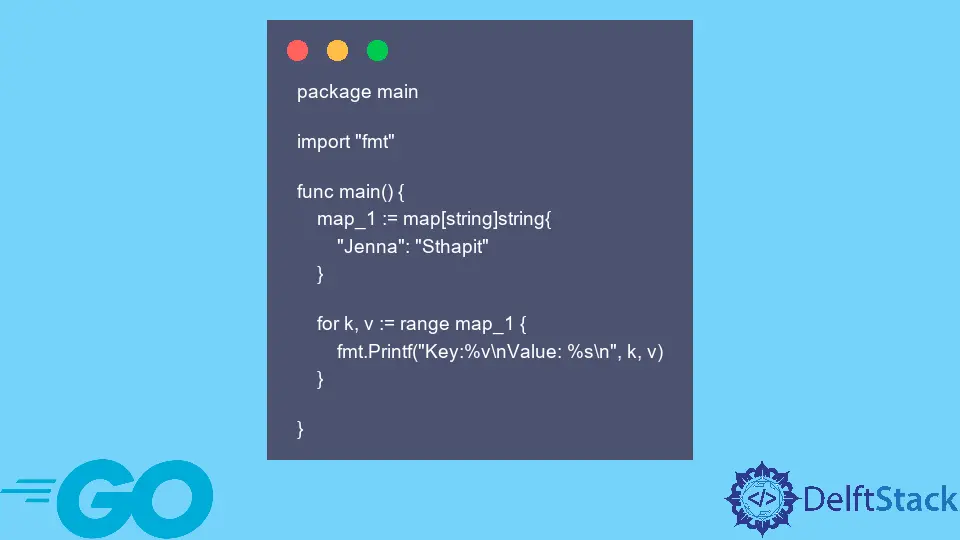
Hash tables are implemented in Go
as map
data type. Go Maps can be viewed as a collection of unordered pairs of key-value pairs. Maps
are one of the most powerful and versatile data types in Go
because of their ability to perform fast lookups, adds, and deletes. We get two return values when we try to get the value of key
in a map
. If the second value is true, then key
is present on the map
.
Structure of map
in Go
Syntax for map
var map_name map[KeyType]ValueType
Where, KeyType
is any comparable datatype
and ValueType
is also any datatype
including map
itself too. The datatype
for all the keys
in a map
must be the same. Similarly, the datatype
for all the values
in a map
must also be same. However, the datatype
of keys
and datatype
of values
can be different.
package main
import "fmt"
func main() {
map_1 := map[string]string{
"Jenna": "Sthapit",
}
for k, v := range map_1 {
fmt.Printf("Key:%v\nValue: %s\n", k, v)
}
}
Output:
Key:Jenna
Value: Sthapit
In the above example, map_1
has string
datatype for both keys
and values
. In map_1
, Sthapit
is value for key Jenna
.
Check for key
in Go-map
Syntax to Check if a map
Contains key
in Go
first_value, second_value := map_name[key_name]
The statement above returns two values viz. first_value
and second_value
. The first_value
gives the value of key
. If the map_name
doesn’t contain key_name
, the first_value
will be the default zero value. Similarly, second_value
is a boolean value which will be true
if the key_name
is present in the map
. We can interpret first_value
and second_value
in different ways to check whether the map_name
contains key_name
.
package main
import "fmt"
func main() {
map_name := map[int]string{
0: "Alok",
1: "Reman",
2: "Riken",
3: "Rudra",
}
_, second_value_1 := map_name[1]
fmt.Printf("second_value for 1: %t\n", second_value_1)
_, second_value_9 := map_name[9]
fmt.Printf("second_value for 9: %t\n", second_value_9)
}
Output:
second_value for 1: true
second_value for 9: false
Here second_value
for 0
is true
as key 0
is present in the map_name
. However, the second_value
for 9
is false
as key 9
is not present in the map_name
.
package main
import "fmt"
func main() {
map_name := map[string]string{
"Giri": "Alok",
"Nembang": "Reman",
"Maharjan": "Riken",
"Jha": "Rudra",
}
if first_value, second_value := map_name["Giri"]; second_value {
fmt.Printf("Giri is present in map. Value is: %s\n", first_value)
} else {
fmt.Printf("Giri is not present in map.\n")
}
if first_value, second_value := map_name["Sthapit"]; second_value {
fmt.Printf("Sthapit is present in map. Value is: %s\n", first_value)
} else {
fmt.Printf("Sthapit is not present in map. \n")
}
}
Output:
Giri is present in map. Value is: Alok
Sthapit is not present in map.
In this example, we used if
statement to check if a key
exists in the map
. Giri
is a key
and Sthapit
is not a key
of map_name
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn