String Interpolation in Go
Musfirah Waseem
Jan 30, 2023
- Specifiers in Go
-
Use the
fmt.Sprintf()
Method - Use the Go Specifier Table
-
Use the
fmt.Printf()
Method
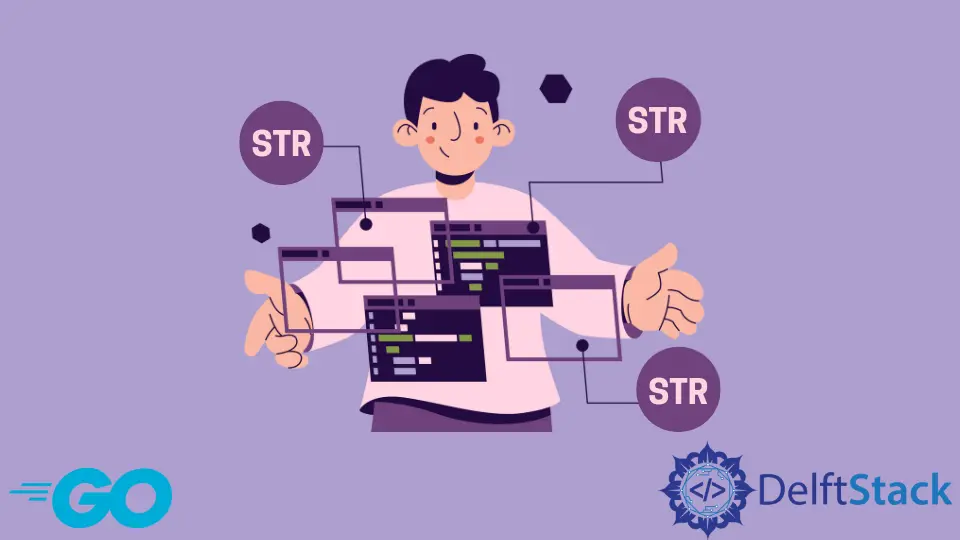
Go has many string interpolation methods that replace a variable’s values with a placeholder inside a string.
Specifiers in Go
Specifier | Description |
---|---|
%s |
print a string |
%d |
print an integer |
%v |
print values of all elements in a defined struct |
%+v |
print the names and values of all elements specified in a struct |
Use the fmt.Sprintf()
Method
package main
import (
"fmt"
"io"
"os"
)
func main() {
const string, val = "Hello,", "GoLang is Fun"
output := fmt.Sprintf("%s World! %s.\n", string, val)
io.WriteString(os.Stdout, output)
}
Output:
Hello, World! GoLang is Fun.
Note that one must import the fmt
package to use this method.
Use the Go Specifier Table
package main
import "fmt"
func main() {
string := "Go language was designed at %s in %d."
place := "Google"
year := 2007
output := fmt.Sprintf(string, place, year)
fmt.Println(output)
}
Output:
Go language was designed at Google in 2007.
The above code snippets display the string literal that contains interpolation expressions.
Use the fmt.Printf()
Method
package main
import "fmt"
func square(i int) int {
return i * i
}
func main() {
fmt.Printf("Square of 2 is %v\n", square(2))
}
Output:
Square of 2 is 4
We have directly interpolated the string in the above code during our output line command.
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn