How to Set Log Levels in Go
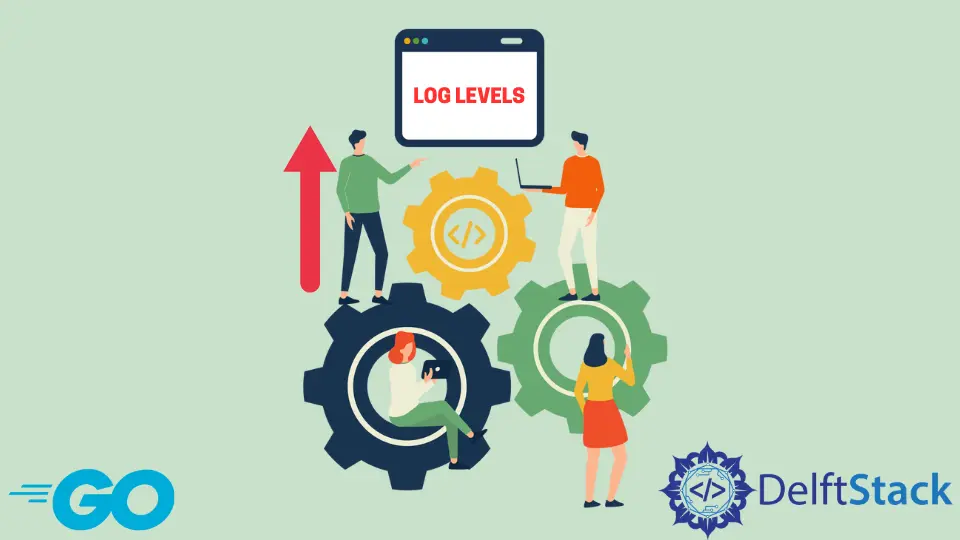
This tutorial demonstrates how to create and use log levels in Golang.
Log Levels in Go
Golang provides a package for logging named log
, which is a simple logging package. This package doesn’t offer leveled logging; if we want leveled logging, we have to manually add prefixes like debug
, info
, and warn
.
Even the Golang log
package doesn’t have the leveled log functionality, but it still provides the basic tools to implement log levels manually. Let’s try a basic example for the Golang log
package:
package main
import "log"
func main() {
log.Println("Hello, This is delftstack.com!")
}
The code above will log the given content and print it. See the output:
2009/11/10 23:00:00 Hello, This is delftstack.com!
But what about log levels? As mentioned above, we can create custom log levels and a custom logger.
We can use the method log.New()
, which takes the following three parameters to create a new logger:
out
- Theout
is used to implement theio.writer
interface to write the log data.prefix
- Aprefix
is a string that will be added to the start of each line.flag
- Theflag
is used to define which properties will be used by the logger.
Now seven log levels are usually used:
Log Level | Description |
---|---|
Trace |
Lowest Level |
Debug |
Second Level |
Info |
Third Level |
Warn |
Fourth Level |
Error |
Fifth Level |
Fatal |
Sixth Level |
Panic |
Highest Level |
Let’s try an example to create custom logging levels based on the Info
, Warning
, Debug
, and Error
levels:
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
var (
Warning_Level *log.Logger
Info_Level *log.Logger
Debug_Level *log.Logger
Error_Level *log.Logger
)
func init() {
file, err := os.OpenFile("Demo_logs.txt", os.O_APPEND|os.O_CREATE|os.O_WRONLY, 0666)
if err != nil {
log.Fatal(err)
}
Info_Level = log.New(file, "INFO: ", log.Ldate|log.Ltime|log.Lshortfile)
Warning_Level = log.New(file, "WARNING: ", log.Ldate|log.Ltime|log.Lshortfile)
Debug_Level = log.New(file, "Debug: ", log.Ldate|log.Ltime|log.Lshortfile)
Error_Level = log.New(file, "ERROR: ", log.Ldate|log.Ltime|log.Lshortfile)
}
func main() {
Info_Level.Println("Loading the application.")
Info_Level.Println("Loading taking time.")
Warning_Level.Println("There is warning.")
Debug_Level.Println("Here is debugging information.")
Error_Level.Println("An Error Occured.")
// read the log file
data, err := ioutil.ReadFile("Demo_logs.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nThe file data is : %s", data)
}
The code above creates custom log levels using the log
package of Golang and writes the log info in a file. See the output:
The file data is : INFO: 2009/11/10 23:00:00 prog.go:32: Loading the application.
INFO: 2009/11/10 23:00:00 prog.go:33: Loading taking time.
WARNING: 2009/11/10 23:00:00 prog.go:34: There is warning.
Debug: 2009/11/10 23:00:00 prog.go:35: Here is debugging information.
ERROR: 2009/11/10 23:00:00 prog.go:36: An Error Occured.
Program exited.
Fatal
and Panic
levels can also cause the termination of a program or application, which is why it is impossible to create these log levels with a built-in log
package. But other than the log
package, a few third-party log packages provide seven built-in log levels. Let’s explore them.
Log Levels in Go With zerolog
The zerolog
is a third-party library for Golang used for structured JSON logging. The zerolog
provides a global logger, which can be used along with the log
subpackage for simple logging.
Along with the simple logging, the zerolog
also provides built-in log levels. There are seven log levels in zerolog
, which include Info
, Warning
, Debug
, Error
, and Trace
.
The zerolog
package is provided on GitHub and can be downloaded in Golang using the following command:
go get -u github.com/rs/zerolog/log
Using the log levels is very simple with zerolog
. See the example below:
package main
import (
"github.com/rs/zerolog"
"github.com/rs/zerolog/log"
)
func main() {
zerolog.SetGlobalLevel(zerolog.InfoLevel)
log.Trace().Msg("Tracing..")
log.Info().Msg("The file is loading.")
log.Debug().Msg("Here is some useful debugging information.")
log.Warn().Msg("There is a warning!")
log.Error().Msg("An Error Occured.")
log.Fatal().Msg("An Fatal Error Occured.")
log.Panic().Msg("This is a panic situation.")
}
The code above will log the information based on the log levels. See the output:
{"level":"info","time":"2009-11-10T23:00:00Z","message":"The file is loading."}
{"level":"warn","time":"2009-11-10T23:00:00Z","message":"There is a warning!"}
{"level":"error","time":"2009-11-10T23:00:00Z","message":"An Error Occured."}
{"level":"fatal","time":"2009-11-10T23:00:00Z","message":"An Fatal Error Occured."}
Program exited.
Log Levels in Go With Logrus
Logrus
is another third-party package for Golang, providing logging in JSON. The Logrus
package is provided on GitHub and can be downloaded using the following command in cmd:
go get "github.com/Sirupsen/logrus"
Logrus
provides the seven log levels, which include Trace
, Debug
, Info
, Warn
, Error
, Fatal
, and Panic
sorted based on the severity. Using these log levels is also similar to the zerolog
where we need to use the subpackage log
.
Let’s try an example with log levels from the Logrus
:
package main
import (
log "github.com/sirupsen/logrus"
)
func main() {
log.SetFormatter(&log.JSONFormatter{})
log.SetLevel(log.DebugLevel)
log.SetLevel(log.TraceLevel)
//log.SetLevel(log.PanicLevel)
log.Trace("Tracing the log info, Lowest level")
log.Debug("Debugging information. Level two")
log.Info("Log Info. Level three")
log.Warn("This is warning. Level Four")
log.Error("An error occured. Level Five")
// Calls os.Exit(1) after logging
log.Fatal("Application terminated. Level Six")
// Calls panic() after logging
log.Panic("Panic Situation. Highest Level.")
}
The code above will log the info based on levels using the Logrus
package. We have to set the levels to show some of the log levels.
See the output:
{"level":"trace","msg":"Tracing the log info, Lowest level","time":"2009-11-10T23:00:00Z"}
{"level":"debug","msg":"Debugging information. Level two","time":"2009-11-10T23:00:00Z"}
{"level":"info","msg":"Log Info. Level three","time":"2009-11-10T23:00:00Z"}
{"level":"warning","msg":"This is warning. Level Four","time":"2009-11-10T23:00:00Z"}
{"level":"error","msg":"An error occured. Level Five","time":"2009-11-10T23:00:00Z"}
{"level":"fatal","msg":"Application terminated. Level Six","time":"2009-11-10T23:00:00Z"}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook