Golang Array of Structs
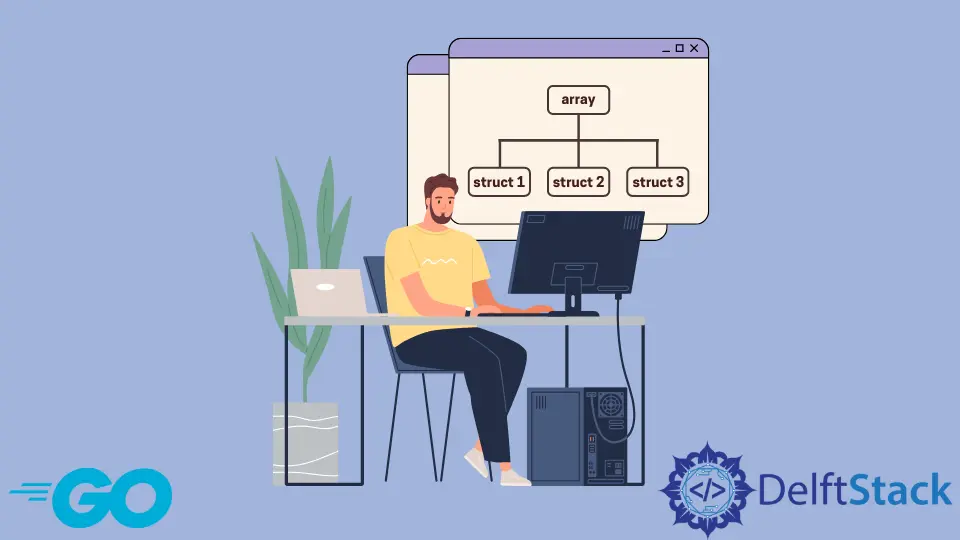
This tutorial demonstrates how to create and use an array of structs in Golang.
Create an Array of Structs in Golang
The struct is considered a user-defined type in Golang, which is used to store different types of data in one type. This concept is usually used in the OOP, where we use a class to store multiple types of data or their properties.
In Golang, we can use the struct, which will store any real-world entity with a set of properties. Golang has the functionality to set the struct of an array.
For example:
type Delftstack struct {
SiteName string
tutorials []tutorial
}
type tutorial struct {
tutorialName string
tutorialId int
tutorialLanguage string
}
The code above shows the type Delftstack
struct uses the slice of type tutorial
struct, where the tutorial
struct is used as an array. These can also be considered nested structs.
Let’s try an example that shows how to use the array of structs in our code:
package main
import "fmt"
type Delftstack struct {
SiteName string
tutorials []tutorial
}
type tutorial struct {
tutorialName string
tutorialId int
tutorialLanguage string
}
func main() {
PythonTutorial := tutorial{"Introduction to Python", 10, "Python"}
JavaTutorial := tutorial{"Introduction to Java", 20, "Java"}
GOTutorial := tutorial{"Introduction to Golang", 30, "Golang"}
tutorials := []tutorial{PythonTutorial, JavaTutorial, GOTutorial}
Delftstack := Delftstack{"Delftstack.com", tutorials}
fmt.Printf("The site with tutorials is %v", Delftstack)
}
The code above initializes a struct Delftstack
and then uses the array of struct tutorial
in the Delftstack
struct. Finally, it prints the site name with the array of tutorials
.
See the output:
The site with tutorials is {Delftstack.com [{Introduction to Python 10 Python} {Introduction to Java 20 Java} {Introduction to Golang 30 Golang}]}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Go Struct
- How to Convert Go Struct to JSON
- How to Print Struct Variables in Console in Go
- How to Convert Struct to String in Golang
- How to Sort Slice of Structs in Go