How to Generate Random String of Fixed Length in Golang
- Understanding Random String Generation
- Using the Math/Rand Package
- Using the Crypto/Rand Package for Secure Strings
- Conclusion
- FAQ
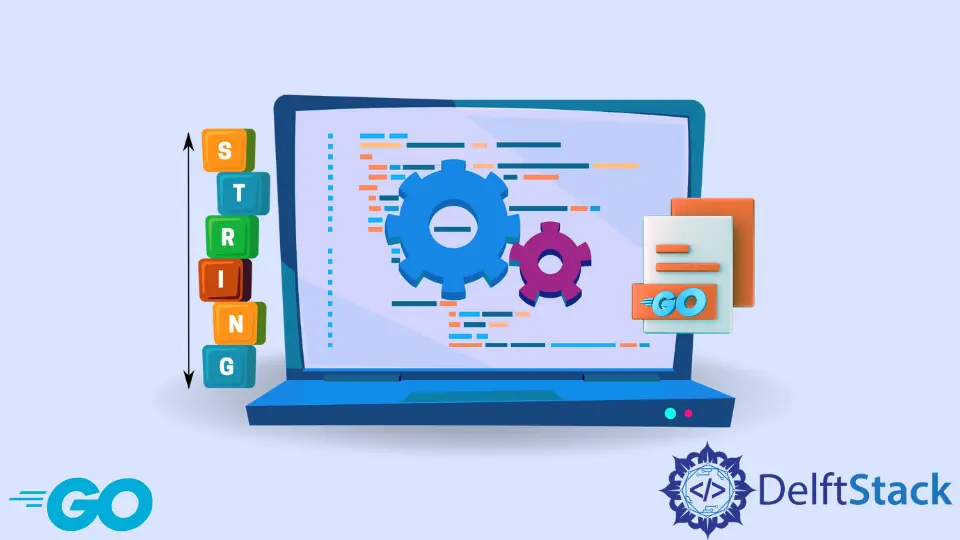
Generating random strings of fixed lengths is a common requirement in programming, especially for tasks like creating unique identifiers, passwords, or tokens.
In this tutorial, we will explore how to generate random strings in Golang, a powerful and efficient programming language. Whether you are a beginner or an experienced developer, this guide will walk you through the process step-by-step. By the end of this article, you will be equipped with the knowledge to generate random strings tailored to your needs. Let’s dive in!
Understanding Random String Generation
Before we jump into the code, it’s essential to understand what we need to accomplish. A random string of fixed length consists of characters selected from a specified set, which can include letters, numbers, and symbols. The flexibility of Golang allows us to create such strings efficiently.
Using the Math/Rand Package
One of the simplest ways to generate a random string in Golang is by using the built-in math/rand
package. This package provides a pseudo-random number generator, which we can utilize to select characters from a predefined set.
Here’s a simple implementation:
package main
import (
"math/rand"
"time"
"fmt"
)
func generateRandomString(length int) string {
const charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
seededRand := rand.New(rand.NewSource(time.Now().UnixNano()))
b := make([]byte, length)
for i := range b {
b[i] = charset[seededRand.Intn(len(charset))]
}
return string(b)
}
func main() {
randomString := generateRandomString(10)
fmt.Println(randomString)
}
Output:
a1B2c3D4e5
In this code, we define a function named generateRandomString
that takes an integer length
as an argument. We create a character set consisting of lowercase and uppercase letters along with digits. The seededRand
variable initializes a new random source based on the current time. We then create a byte slice of the specified length and fill it with random characters selected from the charset. Finally, we convert the byte slice to a string and return it.
Using the Crypto/Rand Package for Secure Strings
If security is a concern, particularly for generating passwords or tokens, you should consider using the crypto/rand
package. This package provides a cryptographically secure random number generator, which is essential for sensitive applications.
Here’s how to implement it:
package main
import (
"crypto/rand"
"fmt"
)
func generateSecureRandomString(length int) (string, error) {
const charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
b := make([]byte, length)
_, err := rand.Read(b)
if err != nil {
return "", err
}
for i := range b {
b[i] = charset[int(b[i])%len(charset)]
}
return string(b), nil
}
func main() {
randomString, err := generateSecureRandomString(10)
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println(randomString)
}
Output:
fG3hJ9kL2m
In this implementation, the generateSecureRandomString
function generates a random byte slice using rand.Read
. We then map each byte to our character set by taking the modulus with the length of the charset. This ensures that the output string consists of valid characters from our predefined set. The function returns both the generated string and any potential error.
Conclusion
Generating random strings of fixed lengths in Golang can be accomplished using different methods depending on your needs. For general use, the math/rand
package is sufficient and easy to implement. However, if your application requires a higher level of security, the crypto/rand
package is the way to go. With the examples provided in this article, you should now be able to generate random strings tailored to your specific requirements. Happy coding!
FAQ
-
How do I generate a random string of a specific length in Golang?
You can use themath/rand
package or thecrypto/rand
package to generate random strings of a specific length by selecting characters from a predefined charset. -
What is the difference between
math/rand
andcrypto/rand
?
Themath/rand
package provides a pseudo-random number generator suitable for non-security-related tasks, whilecrypto/rand
offers a cryptographically secure random number generator for sensitive applications. -
Can I customize the character set used for generating random strings?
Yes, you can customize the character set by modifying thecharset
string in the code examples provided. -
Is it safe to use
math/rand
for generating passwords?
No, it is not recommended to usemath/rand
for generating passwords or tokens due to its predictability. Usecrypto/rand
for secure applications. -
What if I need to generate random strings with special characters?
You can include special characters in thecharset
string to generate random strings that include them.