How to Generate a UUID in Golang
Jay Singh
Feb 02, 2024
-
Generate UUID Using
google/uuid
Package in Golang -
Generate UUID Using
pborman/uuid
Package in Golang
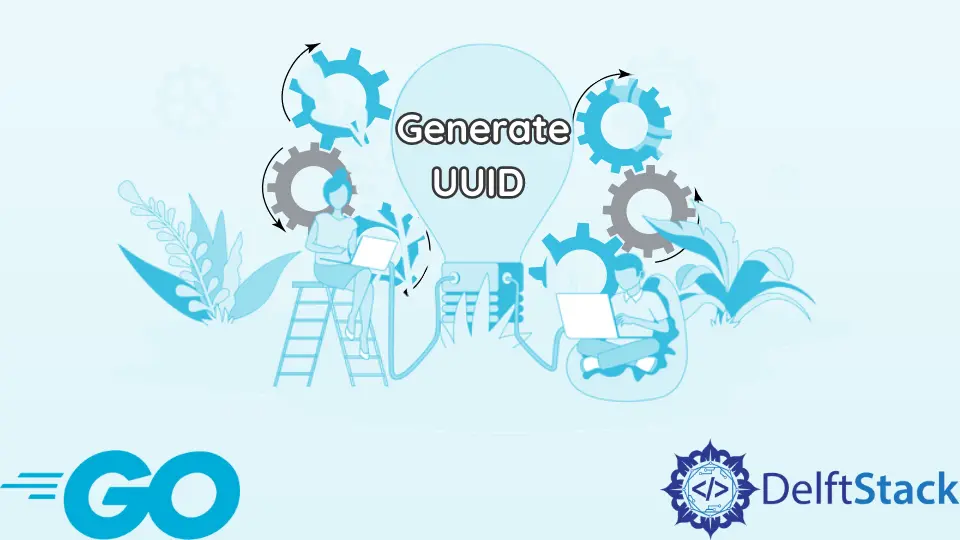
The universal unique identifier (UUID) is a software construction standard usually used to detect information since a duplicate value is near zero. It comprises 32 hexadecimal values that are divided into five blocks.
In this article, you’ll learn how to construct a UUID in Golang.
Generate UUID Using google/uuid
Package in Golang
We will be using the google/uuid
package to generate UUID. Let’s look at the code snippet below.
Example 1:
package main
import (
"fmt"
"github.com/google/uuid"
"strings"
)
func main() {
uuidWithHyphen := uuid.New()
fmt.Println(uuidWithHyphen)
uuid := strings.Replace(uuidWithHyphen.String(), "-", "", -1)
fmt.Println(uuid)
}
Output:
0df82b55-f543-488a-97d2-20f09fd260ff
0df82b55f543488a97d220f09fd260ff
Here is another example of generating a UUID using the google/uuid
package. To begin, you must first install the package.
go get github.com/google/uuid
Then, run the code below.
Example 2:
package main
import (
"fmt"
"github.com/google/uuid"
)
func main() {
uuidValue := uuid.New()
fmt.Printf("%s", uuidValue)
}
Output:
ce547c40-acf9-11e6-80f5-76304dec7eb7
Generate UUID Using pborman/uuid
Package in Golang
Using the pborman/uuid
package, we will generate a UUID.
Example:
package main
import (
"fmt"
"github.com/pborman/uuid"
"strings"
)
func main() {
uuidWithHyphen := uuid.NewRandom()
fmt.Println(uuidWithHyphen)
uuid := strings.Replace(uuidWithHyphen.String(), "-", "", -1)
fmt.Println(uuid)
}
Output:
e995011c-987f-40d2-8f4c-03473b4cdbe0
e995011c987f40d28f4c03473b4cdbe0