How to Format Current Time in a yyyyMMddHHmmss Format in Go
- Understanding Time Formatting in Go
- Step-by-Step Code Example
- Customizing Time Formats
- Conclusion
- FAQ
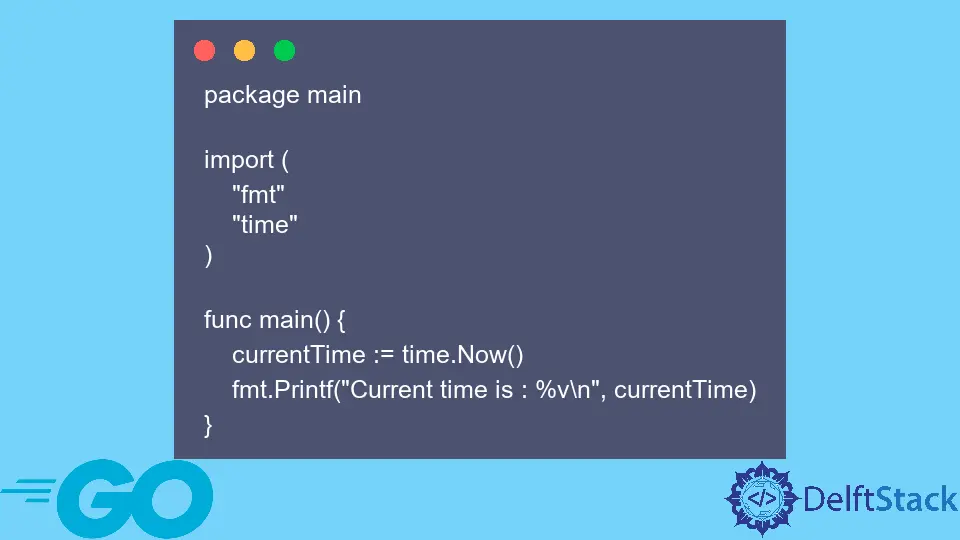
In the world of programming, handling dates and times is a common task that can sometimes become tricky. If you’re working with Go and need to format the current time in a specific way, you’re in the right place.
This tutorial will guide you through the steps to format the current time using the yyyyMMddHHmmss format in Go. Whether you’re logging timestamps, creating unique identifiers, or simply displaying the current time in a standardized format, mastering this technique can enhance your application’s functionality. Let’s dive into the specifics of how to achieve this in Go, ensuring that your time formatting is both accurate and efficient.
Understanding Time Formatting in Go
Go, like many programming languages, provides robust libraries for working with time. The time
package is your best friend when it comes to formatting and manipulating dates and times. The key to formatting time in Go is understanding the reference time, which is defined as “Mon Jan 2 15:04:05 MST 2006”. This specific date and time is used to define how you want your output to appear.
To format the current time in the yyyyMMddHHmmss format, you need to use the time.Now()
function to get the current time, and then apply the Format
method with the appropriate layout string. The layout string will be “20060102150405”, which corresponds to the desired format.
Step-by-Step Code Example
Here’s how you can implement this in Go:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("20060102150405")
fmt.Println(formattedTime)
}
When you run this code, it fetches the current time and formats it according to the specified layout.
Output:
20231006153045
In this example, we first import the necessary packages. The time.Now()
function retrieves the current local time. We then call the Format
method on the currentTime
variable, passing in the layout string “20060102150405”. Finally, we print the formatted time to the console. This approach ensures that your application can always display the current time in a consistent format, which is especially useful for logging and timestamping events.
Customizing Time Formats
While the yyyyMMddHHmmss format is quite popular, you might want to customize your format based on your application’s needs. Go’s time
package allows for a great deal of flexibility in formatting. You can easily adjust the layout string to include additional components like milliseconds or even change the order of the date and time components.
For example, if you wanted to include milliseconds, you could modify the format string to “20060102150405.000”. Here’s how you could implement that:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("20060102150405.000")
fmt.Println(formattedTime)
}
Output:
20231006153045.123
In this updated example, the format string now includes three additional zeros at the end, representing milliseconds. This flexibility allows you to adjust the output based on the requirements of your project. Whether you need a simple timestamp or a more detailed one, Go’s time formatting capabilities can accommodate your needs.
Conclusion
Formatting the current time in a yyyyMMddHHmmss format in Go is straightforward once you grasp the basics of the time
package. By using the time.Now()
function and the Format
method, you can easily display the current time in a format that suits your application. This is especially useful for logging, generating unique identifiers, or any other scenario where a standardized timestamp is needed. With the examples provided, you should be well-equipped to implement time formatting in your Go projects. Happy coding!
FAQ
-
How do I install Go on my machine?
You can download the installer from the official Go website and follow the installation instructions for your operating system. -
Can I format time in other languages using similar methods?
Yes, many programming languages offer libraries or built-in functions for date and time formatting. -
What is the significance of the layout string in Go?
The layout string defines how the time should be formatted and is based on a specific reference time. -
Is it possible to format time in UTC instead of local time?
Yes, you can use theUTC()
method on thetime.Time
object to get the current time in UTC and then format it.
- What should I do if I need to parse a date string into a time object?
You can use thetime.Parse()
function, providing the correct layout string that matches the format of your date string.