The Foreach Loop in Golang
-
Use the
slice
Function to Implement aforeach
Loop in Golang -
Use the
map
Function to Implement aforeach
Loop in Golang
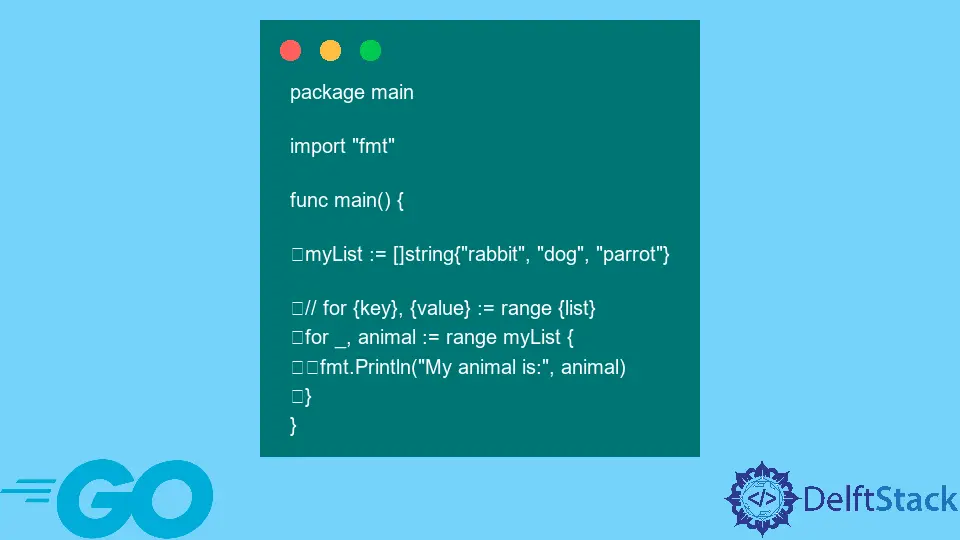
This article will show various examples of implementing a foreach
loop using a function in the Go programming language and a brief discussion about Golang and the used functions.
Use the slice
Function to Implement a foreach
Loop in Golang
The foreach
keyword does not exist in Go; nevertheless, the for
loop can be extended to achieve the same thing.
The distinction is that the range
keyword is used with a for
loop. You can use the slices
key or value within the loop, much like in many other languages foreach
loops.
Example 1:
package main
//import fmt package
import (
"fmt"
)
//program execution starts here
func main() {
//declare and initialize slice
fruits := []string{"mango", "grapes", "banana", "apple"}
//traverse through the slice using for and range
for _, element := range fruits {
//Print each element in new line
fmt.Println(element)
}
}
Output:
mango
grapes
banana
apple
We traverse through a slice of fruit
in the example above. After that, we use for-range
to print each element on a new line.
Example 2:
We print each word by iterating over a string slice
in this example. We use an underscore _
instead of a key since we require the value.
package main
import "fmt"
func main() {
myList := []string{"rabbit", "dog", "parrot"}
// for {key}, {value} := range {list}
for _, animal := range myList {
fmt.Println("My animal is:", animal)
}
}
Output:
My animal is: rabbit
My animal is: dog
My animal is: parrot
Use the map
Function to Implement a foreach
Loop in Golang
An array can iterate and loop over each element in a map
. Golang Maps is a group of key-value pairs that aren’t sorted in any way.
It’s widely used for quick lookups and values that can be retrieved, updated, or deleted using keys.
Example:
package main
import "fmt"
func main() {
myList := map[string]string{
"dog": "woof",
"cat": "meow",
"hedgehog": "sniff",
}
for animal, noise := range myList {
fmt.Println("The", animal, "went", noise)
}
}
Output:
The cat went meow
The hedgehog went sniff
The dog went woof