How to Extract a Substring in Golang
Jay Singh
Feb 02, 2024
- Extract Substring Using Index in Golang
- Extract Single Character Using Simple Indexing in Golang
- Extract Substring Using Range-Based Slicing in Golang
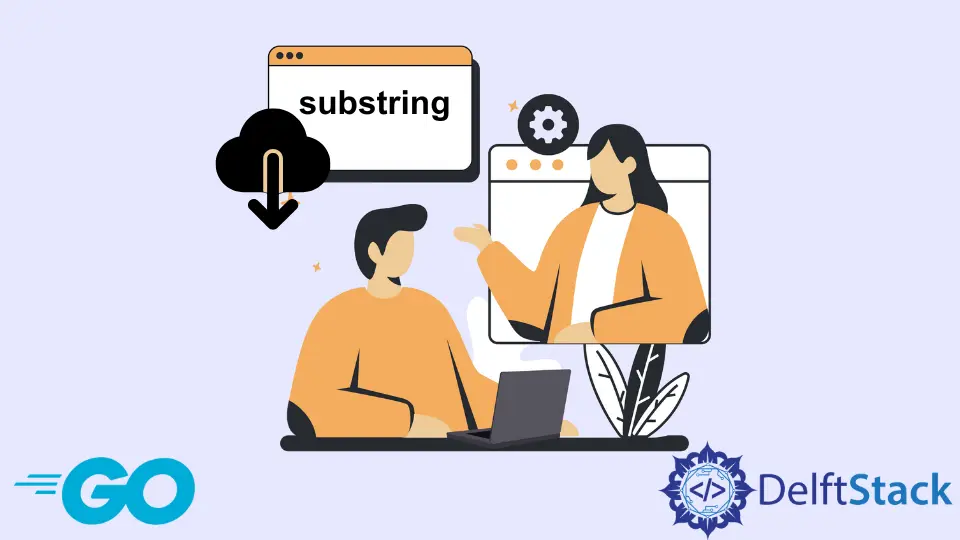
A substring is a collection of characters included inside a larger string set. Most of the time, you’ll need to extract a portion of a string to preserve it for later use.
This article will show us how to extract substring with different methods in Golang.
Extract Substring Using Index in Golang
The index can be used to extract a single character from a string. The text "Hello Boss!"
is used in the following example to extract a substring from index 2
to the end of the string.
Code Snippet:
package main
import (
"fmt"
)
func main() {
str := "Hello Boss!"
substr := str[2:len(str)]
fmt.Println(substr)
}
Output:
llo Boss!
Extract Single Character Using Simple Indexing in Golang
Simple indexing can be used to obtain a single character. You must also convert it to a string as shown in the code; else, the ASCII code is returned.
Code Snippet:
package main
import (
"fmt"
)
func main() {
var s string
s = "Hello Boss!"
fmt.Println(string(s[1]))
}
Output:
e
Extract Substring Using Range-Based Slicing in Golang
Range-based slicing is one of the most efficient techniques to generate a substring in Golang.
Code Snippet:
package main
import (
"fmt"
)
func main() {
s := "Hello Boss!"
fmt.Println(s[1:6])
}
Output:
ello