How to Execute Shell Command in Go
- Execute Shell Command Using the os/exec Package in Go
- Execute Shell Command Using the os/exec Package With Passing Argument in Go
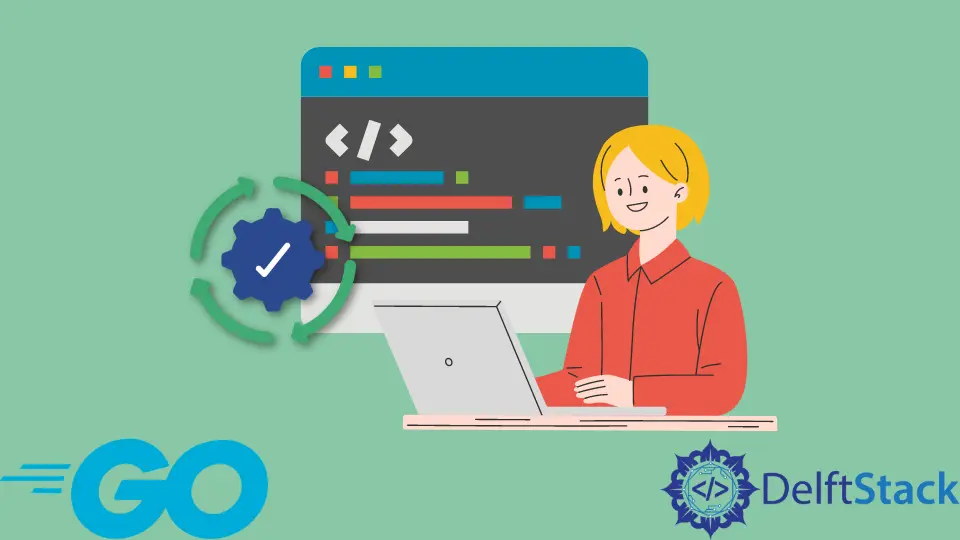
Shell commands tell the system how to do a particular task. External commands are executed via package exec
.
The os/exec package, unlike the system library call from C and other languages, does not execute the system shell and does not expand any glob patterns or handle any shell-like expansions, pipelines, or redirections.
The package is more similar to the exec family of functions in C.
In this article, you’ll learn how to execute shell or external commands in Golang.
Execute Shell Command Using the os/exec Package in Go
Any OS or system command can be triggered using Go’s os/exec package. It provides two functions that can be used to do this: Command, which creates the cmd
object, and Output, which executes the command and returns the standard output.
package main
import (
"fmt"
"log"
"os/exec"
)
func main() {
out, err := exec.Command("pwd").Output()
if err != nil {
log.Fatal(err)
}
fmt.Println(string(out))
}
Output:
It will return the location of your current working directory.
Now, let’s see how we can use the os/exec package to execute simple commands like ls
.
First, we must import the three crucial packages, i.e., fmt, os/exec, and runtime. After that, we’ll construct an execute()
method that will start running the code.
package main
import (
"fmt"
"os/exec"
"runtime"
)
func execute() {
out, err := exec.Command("ls").Output()
if err != nil {
fmt.Printf("%s", err)
}
fmt.Println("Program executed")
output := string(out[:])
fmt.Println(output)
}
func main() {
if runtime.GOOS == "windows" {
fmt.Println("This program is not aplicable for windows machine.")
} else {
execute()
}
}
Output:
Program executed
bin
dev
etc
home
lib
lib64
proc
root
sys
tmp
tmpfs
usr
var
Execute Shell Command Using the os/exec Package With Passing Argument in Go
The Command
function returns a Cmd
struct that can run the supplied program with the specified parameters. The first argument is the program to be performed; the other arguments are the program parameters.
package main
import (
"bytes"
"fmt"
"log"
"os/exec"
"strings"
)
func main() {
cmd := exec.Command("tr", "a-z", "A-Z")
cmd.Stdin = strings.NewReader("Hello Boss!")
var out bytes.Buffer
cmd.Stdout = &out
err := cmd.Run()
if err != nil {
log.Fatal(err)
}
fmt.Printf("New Message: %q\n", out.String())
}
Output:
New Message: "HELLO BOSS!"