Enumerator in Go
-
Use the
iota
to Representenums
in Go -
Use the
iota
to Auto-Increment Number Declaration in Go -
Use the
iota
to Create Common Behavior in Go
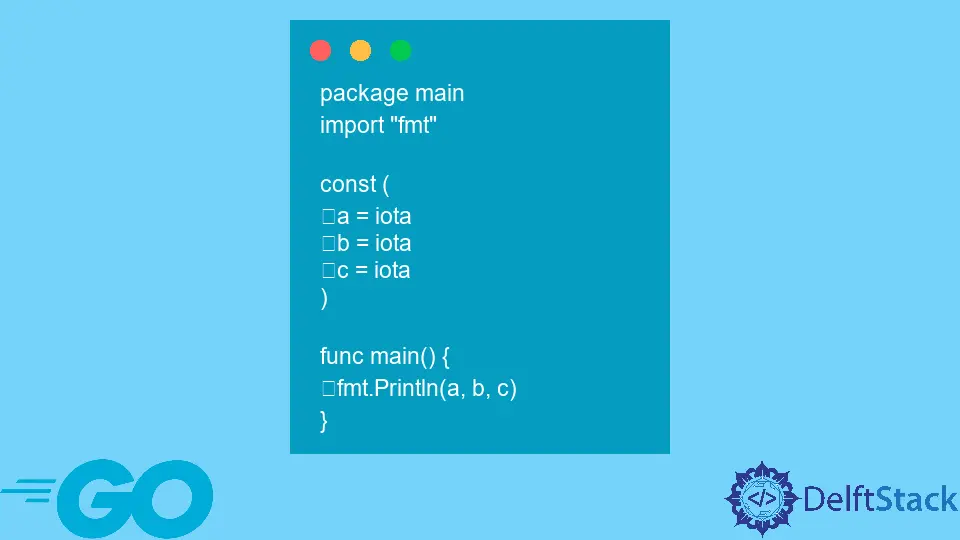
An enum
(short for enumerator) is used to design complicated groupings of constants with meaningful names but simple and distinct values.
In Go, there is no enum
data type. We use the identifier iota
that is predefined, and enums
are not tightly typed.
Use the iota
to Represent enums
in Go
iota
is a constant identifier that can simplify auto-increment number declarations. It denotes a zero-based integer constant.
The iota
keyword represents the numeric constants 0, 1, 2,...
. The term const
appears in the source code resets to 0
and increases with each const
specification.
package main
import "fmt"
const (
a = iota
b = iota
c = iota
)
func main() {
fmt.Println(a, b, c)
}
Output:
0 1 2
Use the iota
to Auto-Increment Number Declaration in Go
Using this method, you can avoid placing sequential iota
in front of each constant.
package main
import "fmt"
const (
a = iota
b
c
)
func main() {
fmt.Println(a, b, c)
}
Output:
0 1 2
Use the iota
to Create Common Behavior in Go
We define a simple enum
named Direction
, which has four potential values: "east"
, "west"
, "north"
and "south"
.
package main
import "fmt"
type Direction int
const (
East = iota + 1
West
North
South
)
// Giving the type a String method to create common behavior
func (d Direction) String() string {
return [...]string{"East", "West", "North", "South"}[d-1]
}
// Giving the type an EnumIndex function allows you to provide common behavior.
func (d Direction) EnumIndex() int {
return int(d)
}
func main() {
var d Direction = West
fmt.Println(d)
fmt.Println(d.String())
fmt.Println(d.EnumIndex())
}
Output:
West
West
2