How to Delete Key From Map in Go
Jay Singh
Feb 02, 2024
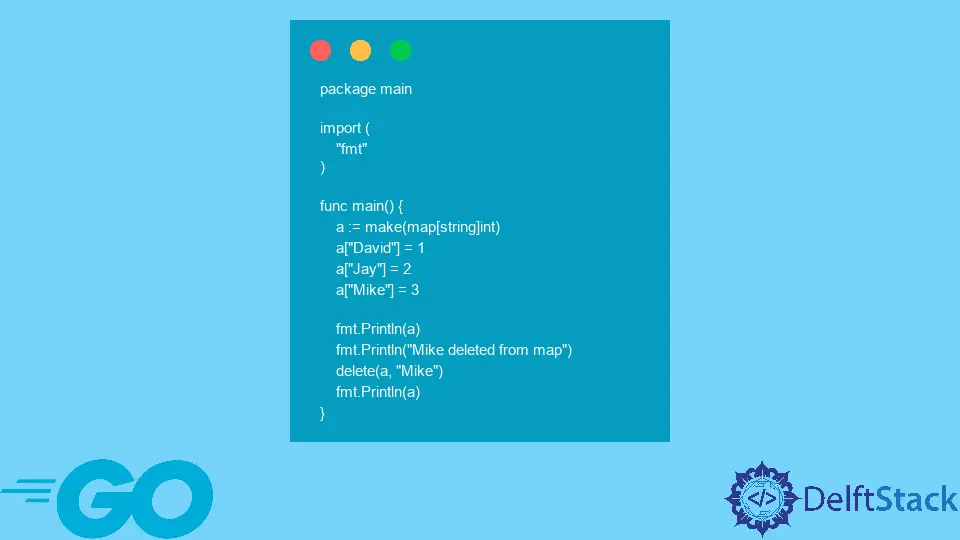
We can use Go’s built-in delete()
function to remove a key from a map. It’s worth noting that when we delete a key from a map, we simultaneously destroy its value since, in Go, the key-value combination is treated as a single object.
Delete Key From Map Using the delete()
Function in Go
We have a map named m in the code example, which has some texts as keys and some integer values as the values of those keys. Later, we use the delete()
method to remove the key Mike
from the map, and then we print the map’s contents once more.
package main
import (
"fmt"
)
func main() {
a := make(map[string]int)
a["David"] = 1
a["Jay"] = 2
a["Mike"] = 3
fmt.Println(a)
fmt.Println("Mike deleted from map")
delete(a, "Mike")
fmt.Println(a)
}
Output:
map[David:1 Jay:2 Mike:3]
Mike deleted from map
map[David:1 Jay:2]
Example 2:
package main
import (
"fmt"
)
func main() {
a := make(map[string]int)
a["David"] = 1
a["Jay"] = 2
a["Mike"] = 3
fmt.Println(a)
fmt.Println("Mike deleted from map")
if _, ok := a["Mike"]; ok {
delete(a, "Mike")
}
fmt.Println(a)
}
Output:
map[David:1 Jay:2 Mike:3]
Mike deleted from map
map[David:1 Jay:2]