How to Copy a Map in Go
- Understanding Maps in Go
- Method 1: Using a Loop to Copy a Map
-
Method 2: Using the
copy
Function - Method 3: Using JSON Marshalling
- Conclusion
- FAQ
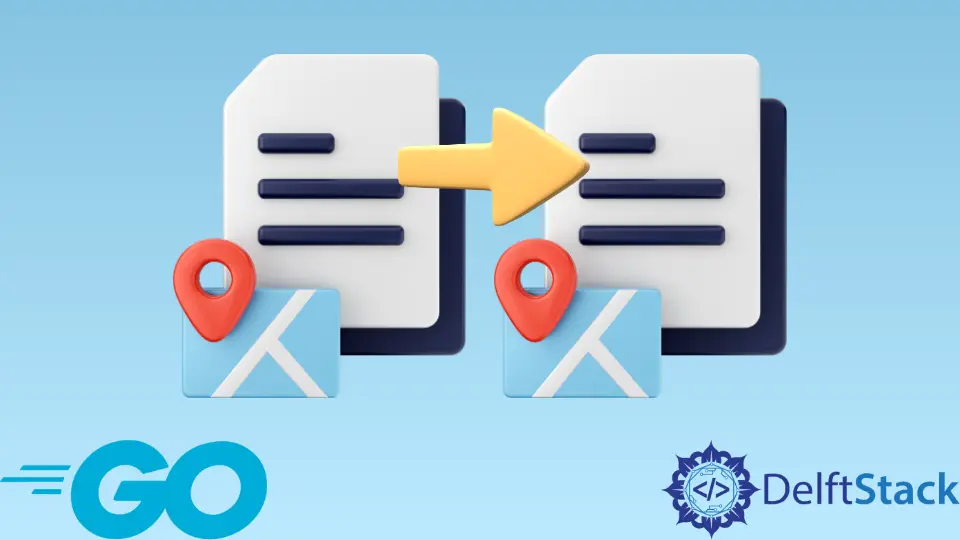
When working with Go (Golang), you may find yourself needing to copy maps frequently. Maps are a fundamental data structure in Go that allow you to store key-value pairs. However, copying a map isn’t as straightforward as it might seem. Unlike other data types, maps in Go are reference types, meaning that when you assign one map to another, they both point to the same underlying data. This can lead to unexpected behavior if you’re not careful.
In this tutorial, we will explore different methods to copy a map in Go, ensuring you understand how to handle this essential task effectively.
Understanding Maps in Go
Before diving into the methods of copying maps, let’s briefly review what maps are in Go. A map is a built-in data type that associates keys with values. Each key must be unique, and the values can be of any data type. Maps are useful for fast lookups and are often used in various applications, from configuration settings to caching data.
Method 1: Using a Loop to Copy a Map
One of the simplest ways to copy a map in Go is by using a loop. This method involves creating a new map and then iterating over the original map to copy each key-value pair. Here’s how you can do it:
package main
import "fmt"
func main() {
originalMap := map[string]int{"a": 1, "b": 2, "c": 3}
copiedMap := make(map[string]int)
for key, value := range originalMap {
copiedMap[key] = value
}
fmt.Println("Original Map:", originalMap)
fmt.Println("Copied Map:", copiedMap)
}
Output:
Original Map: map[a:1 b:2 c:3]
Copied Map: map[a:1 b:2 c:3]
In this code snippet, we first define an original map with some key-value pairs. We then create an empty map called copiedMap
. By using a for
loop, we iterate over each key-value pair in the originalMap
, adding them to the copiedMap
. Finally, we print both maps to verify that the copying was successful. This method is straightforward and works well for small to medium-sized maps.
Method 2: Using the copy
Function
Another method to copy a map in Go is by using the copy
function. However, it’s important to note that the copy
function works specifically with slices, not maps. To use this approach, you would need to convert the map into a slice of key-value pairs, which can then be copied. Let’s see how this can be done:
package main
import "fmt"
func main() {
originalMap := map[string]int{"a": 1, "b": 2, "c": 3}
copiedMap := make(map[string]int)
keys := make([]string, 0, len(originalMap))
for key := range originalMap {
keys = append(keys, key)
}
for _, key := range keys {
copiedMap[key] = originalMap[key]
}
fmt.Println("Original Map:", originalMap)
fmt.Println("Copied Map:", copiedMap)
}
Output:
Original Map: map[a:1 b:2 c:3]
Copied Map: map[a:1 b:2 c:3]
In this approach, we first create a slice called keys
to hold the keys of the original map. We then iterate over the original map to populate this slice. After that, we loop through the keys
slice to copy the corresponding values from the original map into the copiedMap
. This method is slightly more complex but may be useful in specific scenarios, especially when working with large maps.
Method 3: Using JSON Marshalling
A more advanced method for copying a map in Go is by utilizing JSON marshalling. This technique involves converting the map to a JSON string and then unmarshalling it back into a new map. While this method may seem unconventional, it can be particularly useful for deep copying complex maps. Here’s how you can achieve this:
package main
import (
"encoding/json"
"fmt"
)
func main() {
originalMap := map[string]int{"a": 1, "b": 2, "c": 3}
var copiedMap map[string]int
data, _ := json.Marshal(originalMap)
json.Unmarshal(data, &copiedMap)
fmt.Println("Original Map:", originalMap)
fmt.Println("Copied Map:", copiedMap)
}
Output:
Original Map: map[a:1 b:2 c:3]
Copied Map: map[a:1 b:2 c:3]
In this example, we use the encoding/json
package to marshal the original map into a JSON byte slice. We then unmarshal this byte slice into a new map called copiedMap
. This method is particularly effective for deep copying, as it handles nested structures well. However, it does come with a performance overhead due to the conversion to and from JSON.
Conclusion
Copying maps in Go can be accomplished using various methods, each with its own advantages and use cases. Whether you choose to use a simple loop, leverage slices, or employ JSON marshalling, understanding these techniques is crucial for effective programming in Go. By mastering these methods, you can handle maps with confidence and avoid common pitfalls associated with reference types. Remember, the method you choose should align with your specific needs and the complexity of the data you are working with.
FAQ
-
How do I copy a map in Go?
You can copy a map in Go using a loop, slices, or JSON marshalling. -
Is there a built-in function to copy maps in Go?
No, Go does not have a built-in function to copy maps. You need to implement it manually.
-
Can I copy nested maps in Go?
Yes, you can copy nested maps using JSON marshalling for deep copies. -
What happens if I assign one map to another in Go?
Both maps will point to the same underlying data, leading to unintended modifications. -
Is JSON marshalling the best way to copy maps?
It is effective for deep copies but may introduce performance overhead. Use it when necessary.