How to Convert String to Int64 in Go
- Understanding ParseInt() Function
- Basic Example of String to Int64 Conversion
- Handling Errors During Conversion
- Converting Hexadecimal Strings to Int64
- Conclusion
- FAQ
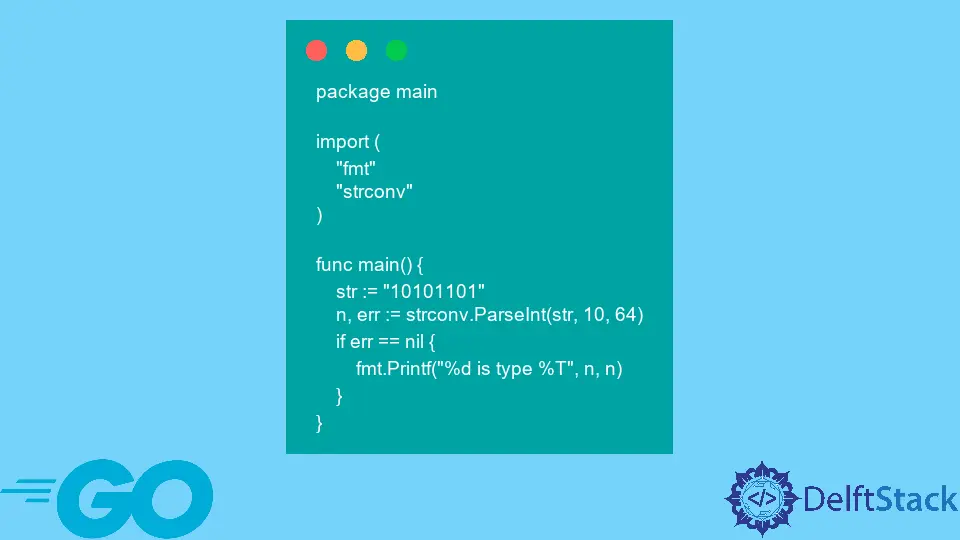
Converting a string to an int64 in Go is a common task that many developers encounter. Whether you’re processing user input, reading data from a file, or handling JSON, you may find yourself needing to convert string representations of numbers into their integer counterparts. The Go programming language provides a straightforward way to accomplish this using the strconv
package.
In this tutorial, we’ll explore how to use the ParseInt()
function to convert strings to int64 values effectively. By the end of this article, you’ll have a solid understanding of how to perform these conversions in your Go applications.
Understanding ParseInt() Function
The ParseInt()
function from the strconv
package is the go-to method for converting strings to integers in Go. This function allows you to specify the base of the number system you’re working with, making it versatile for various applications. The function signature looks like this:
func ParseInt(s string, base int, bitSize int) (i int64, err error)
s
: The string to be converted.base
: The base for conversion (0 for auto-detection).bitSize
: The bit size of the integer type (0 to 64 for int64).
Using this function, you can convert a string to an int64 by simply passing the appropriate parameters.
Basic Example of String to Int64 Conversion
Let’s start with a simple example where we convert a string representing a number into an int64. Here’s how you can do it:
package main
import (
"fmt"
"strconv"
)
func main() {
strNum := "12345"
intNum, err := strconv.ParseInt(strNum, 10, 64)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Converted int64:", intNum)
}
}
In this code snippet, we define a string strNum
holding the numeric value “12345”. We then call strconv.ParseInt()
with the string, specifying base 10 and a bit size of 64. If the conversion is successful, we print the converted int64 value. If there’s an error, we handle it gracefully by printing the error message.
Output:
Converted int64: 12345
The ParseInt()
function works seamlessly, converting the string to an int64 type. Error handling is crucial here, as it helps us catch any issues that may arise during conversion, such as if the string contains non-numeric characters.
Handling Errors During Conversion
When converting strings to int64, it’s essential to handle potential errors gracefully. Strings that do not represent valid integers will trigger an error during conversion. Here’s how you can manage such scenarios:
package main
import (
"fmt"
"strconv"
)
func main() {
strNum := "abc123"
intNum, err := strconv.ParseInt(strNum, 10, 64)
if err != nil {
fmt.Println("Conversion failed:", err)
} else {
fmt.Println("Converted int64:", intNum)
}
}
In this example, we attempt to convert the string “abc123” into an int64. Since this string is not a valid integer, ParseInt()
will return an error. We check for this error and print a message indicating that the conversion failed.
Output:
Conversion failed: strconv.ParseInt: parsing "abc123": invalid syntax
This practice of error handling is vital in production code, ensuring that your application can respond appropriately to unexpected input.
Converting Hexadecimal Strings to Int64
Another powerful feature of ParseInt()
is its ability to convert strings representing numbers in different bases, such as hexadecimal. This can be particularly useful in applications dealing with low-level data manipulation or networking. Here’s an example:
package main
import (
"fmt"
"strconv"
)
func main() {
hexStr := "1a"
intNum, err := strconv.ParseInt(hexStr, 16, 64)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Converted int64 from hex:", intNum)
}
}
In this code, we convert the hexadecimal string “1a” into an int64. By specifying base 16 in the ParseInt()
function, we indicate that the input string is in hexadecimal format.
Output:
Converted int64 from hex: 26
This example demonstrates how flexible the ParseInt()
function is, allowing you to handle various numeral systems easily.
Conclusion
Converting strings to int64 in Go is a straightforward process, thanks to the strconv.ParseInt()
function. By specifying the correct base and bit size, you can easily convert strings representing integers or even hexadecimal values. Remember to handle errors gracefully to ensure your application runs smoothly, even when faced with unexpected input. With these techniques in your toolkit, you’ll be well-equipped to handle string-to-int64 conversions in your Go projects.
FAQ
-
How can I convert a string representing a decimal number to int64 in Go?
You can usestrconv.ParseInt()
with base 10 to convert a decimal string to int64. -
What happens if the string contains non-numeric characters?
If the string has non-numeric characters,ParseInt()
will return an error indicating invalid syntax. -
Can I convert a hexadecimal string to int64 in Go?
Yes, you can specify base 16 inParseInt()
to convert hexadecimal strings to int64. -
Is it necessary to handle errors while converting strings to int64?
Yes, error handling is crucial to ensure your application can manage unexpected input gracefully. -
What is the maximum value for int64 in Go?
The maximum value for int64 is 9223372036854775807.