How to Composite Literals in Go
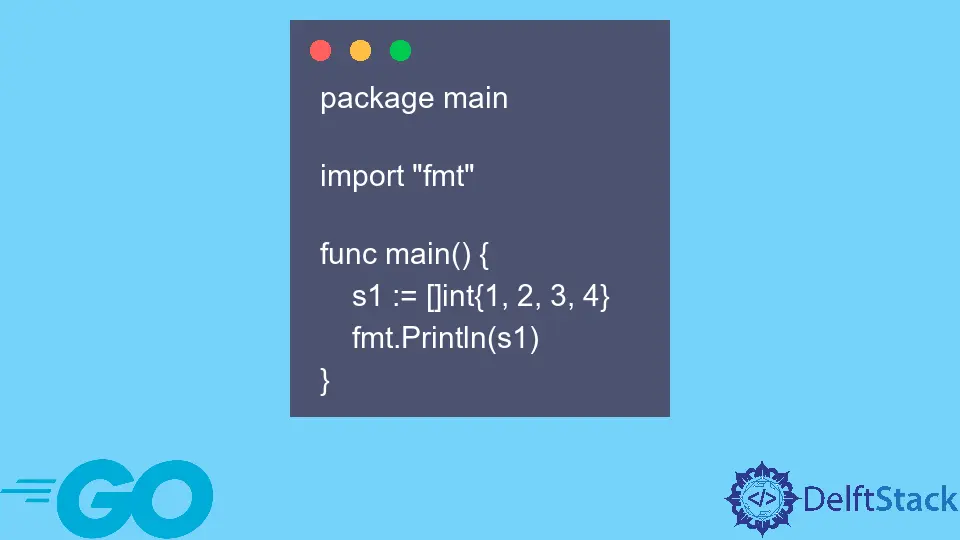
Go has various built-in identifier types, also known as predeclared types, such as boolean, string, numeric (float32, float64, int, int8, int16, int32, complex), etc.
There are also composite kinds, which consist of predeclared types. Values for arrays, structs, slices, and maps are constructed using composite literal.
Each time they are assessed, a new value is created. It consists of the literal’s type followed by a brace-bound list of items.
Let’s look at some composite literal instances.
Slice Composite Literals in Go
Slice is a composite data type that works similarly to an array in that it holds items of the same data type. The critical distinction between an array and a slice is that a slice can change size dynamically, whereas an array cannot.
package main
import "fmt"
func main() {
s1 := []int{1, 2, 3, 4}
fmt.Println(s1)
}
Output:
[1 2 3 4]
Array Composite Literals in Go
When one map is assigned to another, the two maps share all (underlying) components. Adding items to (or removing elements from) one map will affect the other.
If a slice is assigned to another, the two will share all (underlying) components, just as map assignments. Their relative lengths and capacity are equal.
If the length or capacity of one slice changes later, the change does not reflect in the other slice. All items are copied from the source array to the destination array when one is assigned to another.
The two arrays share no elements.
package main
import "fmt"
func main() {
m0 := map[int]int{0: 2, 1: 3, 2: 4}
m1 := m0
m1[0] = 4
fmt.Println(m0, m1)
s0 := []int{5, 6, 7}
s1 := s0
s1[0] = 4
fmt.Println(s0, s1)
a0 := [...]int{5, 6, 7}
a1 := a0
a1[0] = 4
fmt.Println(a0, a1)
}
Output:
map[0:4 1:3 2:4] map[0:4 1:3 2:4]
[4 6 7] [4 6 7]
[5 6 7] [4 6 7]