How to Check if a Slice Contains an Element in Golang
Jay Singh
Feb 02, 2024
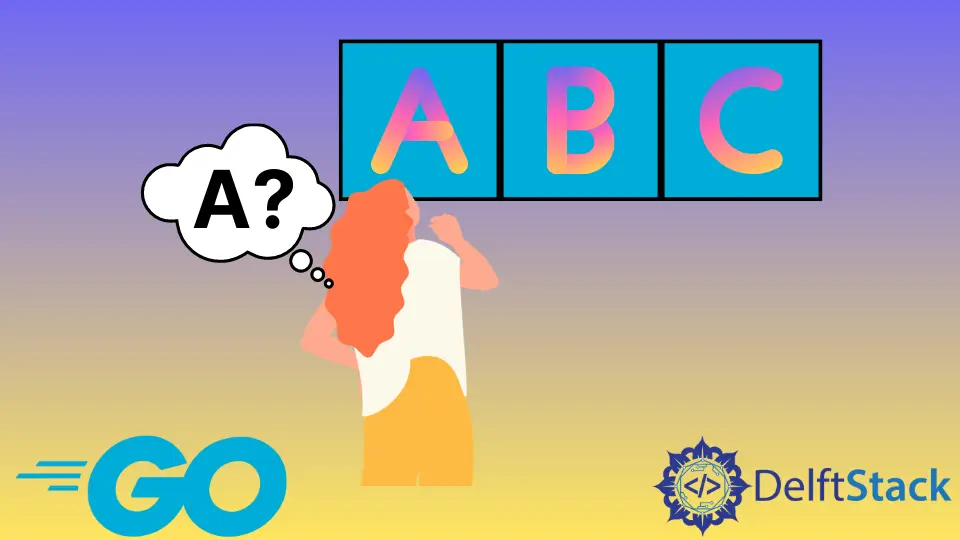
Go lacks a language concept or a standard library function for determining if a given value belongs in a slice. You’ll need to create your own contains()
function with two parameters, the slice and the element you’re looking for.
We will learn in this tutorial how to use Golang to determine whether an element is present or not.
Use the contains()
Function to Check an Element Is Present or Not in Slice
In this following example, contains()
is used to check if an element is present or not in the slice.
Example 1:
package main
import "fmt"
func contains(elems []string, v string) bool {
for _, s := range elems {
if v == s {
return true
}
}
return false
}
func main() {
fmt.Println(contains([]string{"a", "b", "c"}, "b"))
fmt.Println(contains([]string{"a", "b", "c"}, "d"))
}
Output:
true
false
In the next example, we take a slice of integers in slice1
. This slice is examined to see if the element 5
is present.
Example 2:
package main
import "fmt"
func main() {
slice1 := []int{1, 2, 3, 4, 5}
var element int = 5
var result bool = false
for _, x := range slice1 {
if x == element {
result = true
break
}
}
if result {
fmt.Print("Element is present.")
} else {
fmt.Print("Element is not present.")
}
}
Output:
Element is present.
The last example contains a slice of names in an array called s
and checks if "Rick"
and "Mike"
are present or not.
Example 3:
package main
import "fmt"
func contains(s []string, str string) bool {
for _, v := range s {
if v == str {
return true
}
}
return false
}
func main() {
s := []string{"Jay", "Rick", "Morty", "Jerry"}
fmt.Println(contains(s, "Rick"))
fmt.Println(contains(s, "Mike"))
}
Output:
true
false