How to Check if a File Exists or Not in Go
Jay Singh
Feb 02, 2024
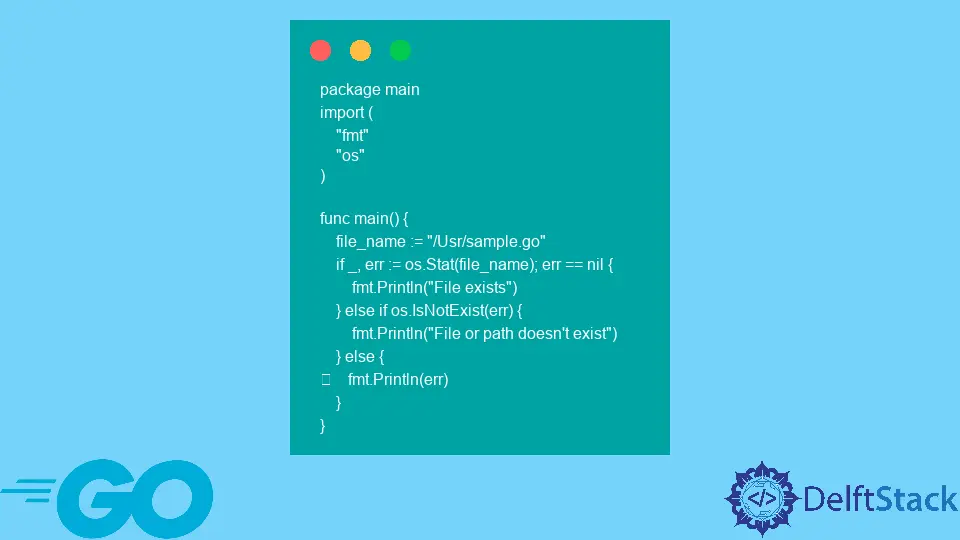
This article will discuss checking if a file exists or not in Go.
Use IsNotExist()
and Stat()
to Check if the File Exists or Not in Go
We use the IsNotExist()
and Stat()
methods from the os
package in the Go programming language to determine whether a file exists.
The Stat()
function returns an object that contains information about a file. If the file does not exist, it produces an error object.
Below are examples of code using IsNotExist()
and Stat()
.
Example 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
Output:
demo.txt file exist
demo.csv file does not exist
Example 2:
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
Output:
File or path doesn't exist