How to Cast Interface Into Concrete Type in Go
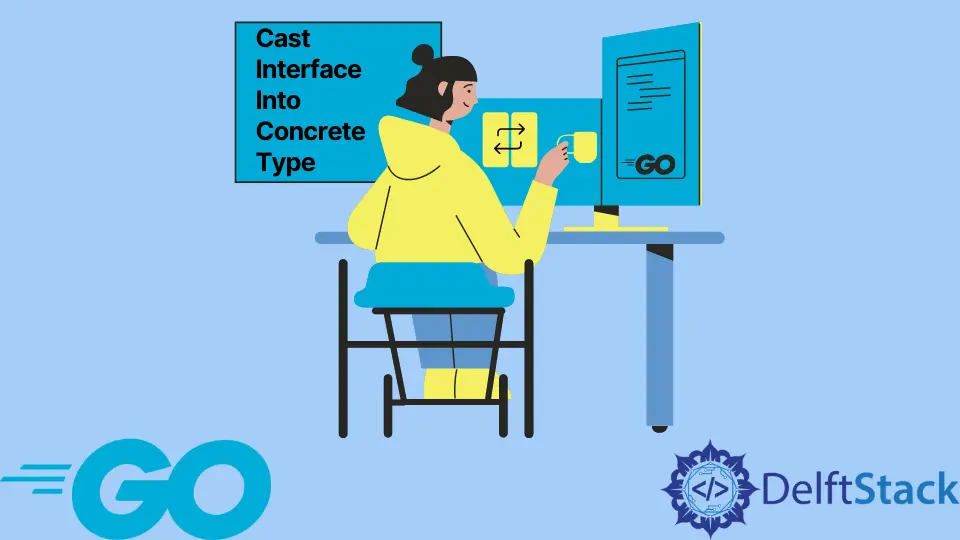
Casting an interface into a concrete type in Go can seem daunting at first, but once you grasp the underlying principles, it becomes a straightforward process.
In this tutorial, we will delve into the nuances of type assertion and type switches, two essential techniques for handling interfaces in Go. By the end of this article, you will have a clear understanding of how to cast interfaces into concrete types effectively, enhancing your Go programming skills. Whether you are a beginner or an experienced developer, this guide will provide you with valuable insights and practical examples to help you master this concept. Let’s get started!
Understanding Interfaces in Go
Before we dive into the casting process, let’s take a moment to understand what an interface is in Go. An interface defines a contract that a type must adhere to, allowing for polymorphism. It essentially allows different types to be treated as the same type if they implement the same methods. This flexibility is one of Go’s powerful features, enabling developers to write more generic and reusable code. However, when you work with interfaces, there might be times when you need to convert an interface back into its concrete type. This is where type assertion and type switches come into play.
Type Assertion
Type assertion is a method that allows you to extract the concrete type from an interface. It’s a simple yet powerful technique in Go. Here’s how you can use type assertion to cast an interface to a concrete type.
package main
import (
"fmt"
)
type Animal interface {
Speak() string
}
type Dog struct{}
func (d Dog) Speak() string {
return "Woof!"
}
func main() {
var animal Animal = Dog{}
dog, ok := animal.(Dog)
if ok {
fmt.Println("The dog says:", dog.Speak())
} else {
fmt.Println("The assertion failed.")
}
}
Output:
The dog says: Woof!
In this example, we define an interface Animal
with a method Speak
. We then create a struct Dog
that implements this interface. In the main
function, we assign a Dog
instance to the animal
variable of type Animal
. The type assertion animal.(Dog)
attempts to cast the interface back to its concrete type, Dog
. The ok
variable indicates whether the assertion was successful. If successful, we call the Speak
method on the dog
variable, which outputs “Woof!”. If the assertion fails, we print an error message.
Type Switch
A type switch is a more versatile way to handle multiple types stored in an interface. It allows you to check the type of an interface and perform different actions based on that type. Here’s how you can implement a type switch in Go.
package main
import (
"fmt"
)
type Animal interface {
Speak() string
}
type Cat struct{}
func (c Cat) Speak() string {
return "Meow!"
}
func main() {
var animal Animal = Cat{}
switch a := animal.(type) {
case Dog:
fmt.Println("The dog says:", a.Speak())
case Cat:
fmt.Println("The cat says:", a.Speak())
default:
fmt.Println("Unknown animal.")
}
}
Output:
The cat says: Meow!
In this code, we define another struct Cat
that also implements the Animal
interface. In the main
function, we use a type switch to determine the concrete type of the animal
variable. The switch
statement checks the type of a
, and based on its type, it calls the appropriate Speak
method. If animal
is of type Dog
, it will print the dog’s sound; if it is of type Cat
, it will print the cat’s sound. If it doesn’t match any case, it defaults to printing “Unknown animal.” This approach is particularly useful when you have multiple types that implement the same interface.
Conclusion
Casting interfaces into concrete types in Go is an essential skill that enhances your ability to work with polymorphic data structures. By mastering type assertions and type switches, you can write more flexible and maintainable code. Remember, interfaces allow you to define behaviors without specifying the exact types, making your code more adaptable. Keep practicing, and soon, casting interfaces will become second nature.
FAQ
-
What is an interface in Go?
An interface in Go is a type that defines a contract for methods that a concrete type must implement. -
How do I check if a type assertion is successful?
You can check if a type assertion is successful by using the comma-ok idiom, which returns a boolean indicating the success of the assertion. -
What is the difference between type assertion and type switch?
Type assertion is used to extract a single concrete type from an interface, while a type switch allows you to handle multiple types stored in an interface. -
Can I use type assertion on any interface?
Yes, you can use type assertion on any interface as long as the concrete type implements the interface’s methods. -
What happens if a type assertion fails?
If a type assertion fails, it will cause a panic unless you use the comma-ok idiom to safely check for success.