How to Undo Pushed Commits in Git With Reset and Revert
-
Undo Pushed Commits With the
git reset
Command -
Undo Pushed Commits With the
git revert
Command -
Undo Pushed Commits With the
git checkout
Command
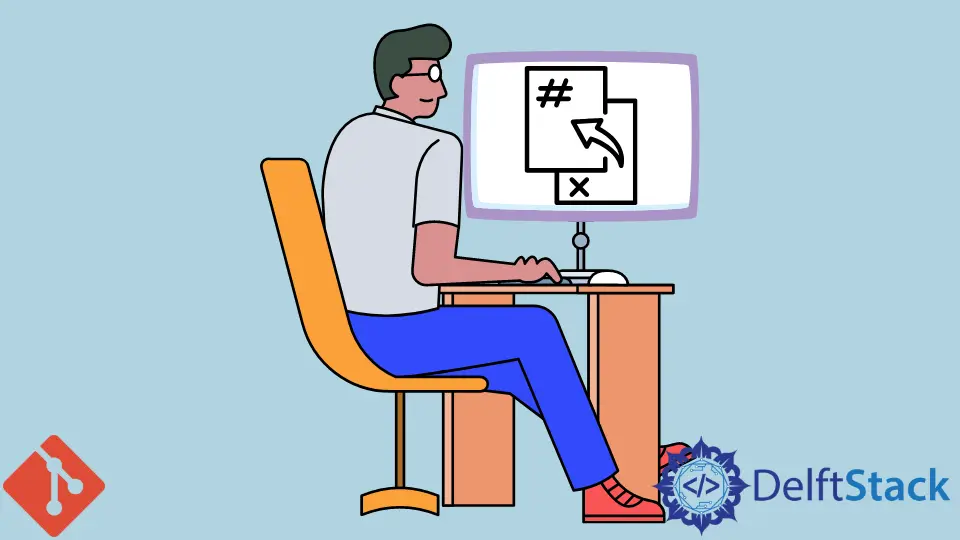
We show three methods to undo pushed commits from a remote repository in Git. We use the git reset
, revert
, and checkout
commands for this.
When we use git reset
, we also remove any trace of the unwanted commits from the repository history. But with git revert
, both the original and undo commits remain in history.
If we use git checkout
, we undo the changes in a new branch.
Undo Pushed Commits With the git reset
Command
We create a undo_pushed_commits_local
repository and fill it with a few healthy (good) commits.
mkdir undo_pushed_commits_local
git init
Then add/modify files.
git add --all
git commit -m "Make healthy commit"
Add/modify more files.
git add -all
git commit -m "Make another healthy commit"
We will now push these commits to the undo-pushed-commits-remote
repository on GitHub.
git remote add undo-remote git@github.com:danielturidandy/undo-pushed-commits-remote.git
git push undo-remote master
Our remote repository now has two commits.
Now, let us make the bad commit.
echo "This is a bad addition" >> file1.txt
echo "This is a bad addition" >> file2.txt
git add -all
git commit -m "Make a bad commit"
We push this commit to the remote repo.
git push undo-remote
Our remote has the bad commit:
We now reset
to the last good commit in our local repo. We use the --hard
option to remove any trace of the bad commits from the commit history.
git log
git reset --hard <SHA of the last good commit>
We push
the local to the remote repository with the -f option to force the push.
git push -f undo-remote
This method works best with private repositories or repositories with small teams.
Large teams might face issues if some developers pull the remote to their local environment after pushing the bad commits before we reset to a good state. They have no commits history with information of the bad commits to correct their repositories.
Undo Pushed Commits With the git revert
Command
We have here pushed four bad commits to the remote repo.
We can use revert
to undo either a single bad commit or a range of bad commits.
revert
makes a new commit that reverses the unwanted commit. Both the original and reversed commits stay in the repository history.
Undo a Single Pushed Commit With revert
git revert <SHA of the commit we want to revert>
We now push this change into the remote repo. Remember to use the -f flag to make sure no conflicts arise.
git push -f undo-remote
Our remote repository now has the new commit that reverses the bad commit.
Undo a Range of Pushed Commits With revert
git revert <SHA of the oldest commit to revert>..<SHA of the newest commit to revert>
This will revert all the commits in the range provided (Not including the oldest commit, but this may also depend on the version/platform you use.)
We now push the changes to the remote with the -f flag.
git push -f undo-remote
This method is great for public repositories with large teams working on them. As both the bad and the undo commits remain in history, developers can keep their local repositories updated with all the bad pushes/reverts.
Undo Pushed Commits With the git checkout
Command
This technique is a quick hack that forks a new branch without the bad commits. It is great for small codebases and if you are short on time.
We first checkout
to the last good commit.
git checkout <SHA of last known good commit>
We then reach a detached HEAD state at last known good commit in the repository history.
We here now fork a new branch from this state.
git checkout -b branch_without_badcommits
We then push it into the remote into a new branch.
git push -f undo-remote branch_without_badcommits
The remote repository has a new branch now without any bad commits.