How to Undo Git Pull
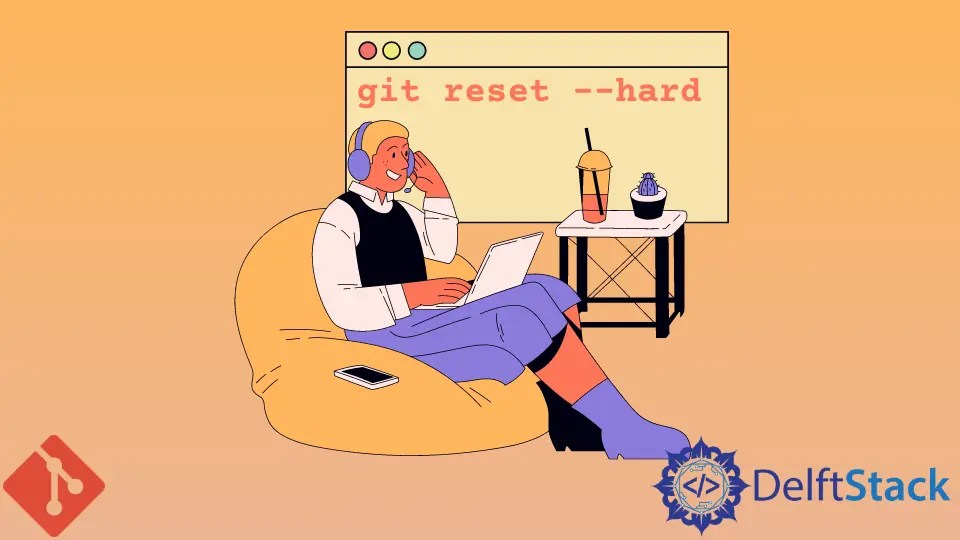
This tutorial demonstrates undoing a git pull to bring a git repository to a previous state using git hard reset.
Undo Git Pull
To undo a git pull with the hard reset, we use the git reset --hard
command and specify the HEAD
.
Let us see the commits that we have made on our git repository by using the git log
command with --oneline
and --graph
options as shown below.
We have made three commits to the repository, and the most recent commit is the * bdb9fc2
.
To undo the recent commit using hard reset, we use the git reset
command with the --hard
option, as shown below. The HEAD^
specifies that return to the commit before HEAD.
The output of the git log
command shows that we have returned to the previous commit.
As shown below, we can also use HEAD~1
to specify to return to the commit before HEAD
. HEAD~2
means a return to two commits before HEAD
.