How to Untrack Folder in Git
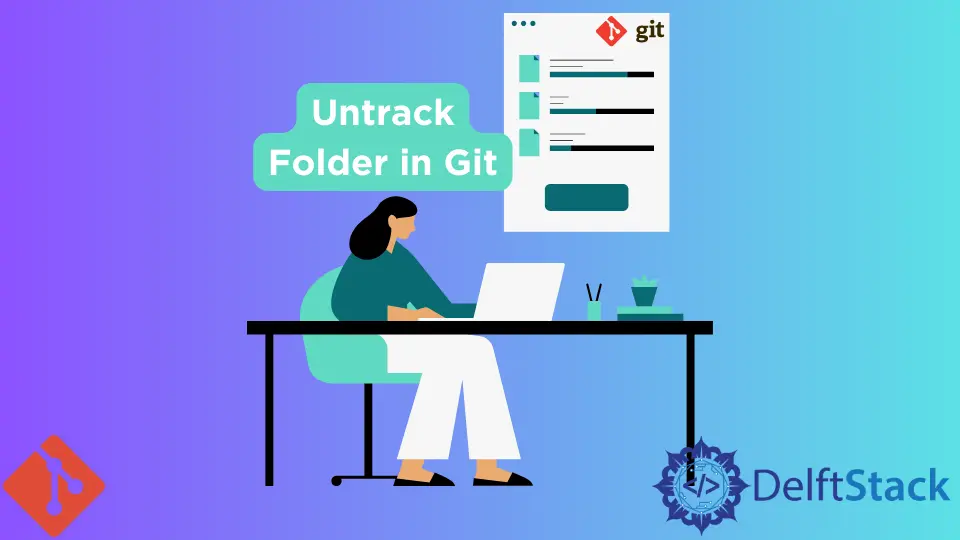
We can untrack the history created for the push or pull commands; we can untrack using the Git command. Git has two options in which files or folders may be tracked or untracked in its working directory.
We will see the difference between tracked or untracked folders in Git below.
Difference Between Tracked and Untracked Files in Git
Tracked files:
The files added and committed to Git
in the last commit, Git knows about it, are called Tracked files. These files can be staged, modified, or not.
Untracked files:
For untracked files, we can say that untracked files are the opposite of tracked files. All the other files in the Git working folder are untracked.
These files are recently added and committed to Git and are ready to be staged to the Git repository, and Git does not know of it.
To check whether the file is tracked or untracked, we will use the command git status
. When we work on various projects, we sometimes encounter situations where we are messed up with unwanted files generated automatically or mistakenly created.
For this situation, either we can .gitignore
these files or remove them through git rm
.
The better option is to go with git rm
because it will clean our repository. This option can remove files or folders individually or in bulk as per our needs.
It can remove files or folders from the index and the working directory. Therefore, this article will explain untracking folders in Git, which is super easy with some small commands.
Untrack Folder in Git
To untrack the folder in Git, we can proceed through the following steps.
-
Commit all changes
Before using the command
git rm
, we should ensure that all our changes are committed, including the.gitignore
file. -
Remove the folder from the repository
As mentioned below, we will accomplish it with the recursive option when we want to untrack a whole folder.
git rm -r --cached <folder>
rm
is an abbreviation of the remove command.-r
will be used for recursive removal.--cached
will remove the folder from the index. Don’t worry; our files are still there and safe.<folder>
will specify the folder we want to remove.
-
Re-adding the untrack folder
We can re-add that folder using the following command.
git add.
-
Commit
The last step, after that, our repository, will be clean.
git commit -m ".gitignore fix"
By pushing the changes to the remote, we can effectively see the changes in the remote repository.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn