How to Undo a Git Pull
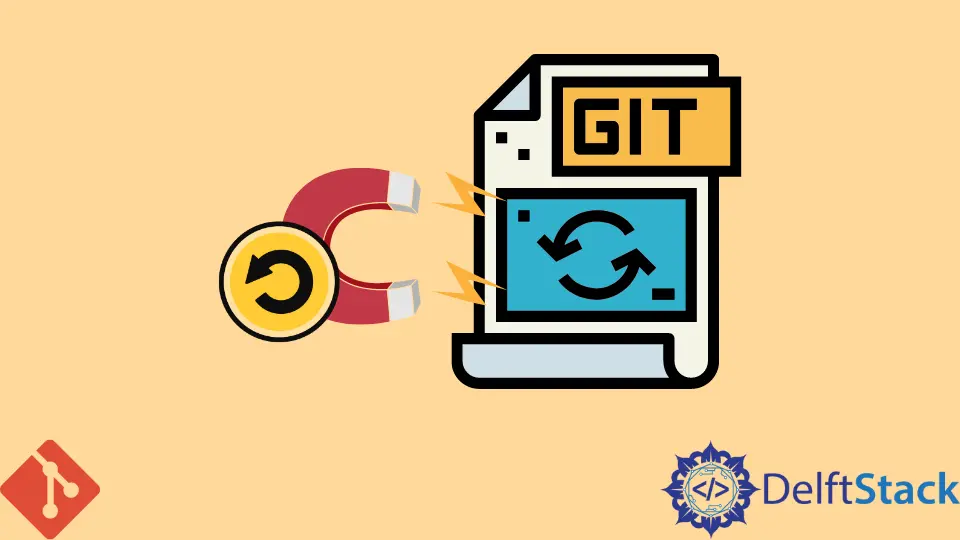
This article illustrates how you can undo the effects of the git pull
command. You may find yourself in a situation where a git pull
command has changed files in your repository, but you would like to restore them to their previous state.
This article will guide you on taking steps to revert your repository.
Undo a Git Pull
The git pull
command combines the git fetch
and git merge Fetch_HEAD
commands. It fetches changes from the remote repository and merges them into the current local branch.
Whatever is at the tip of the remote branch will be merged with your local branch.
If you run the git log --oneline
command, you should see the new commit from the remote repository. At this point, your repo is at HEAD
.
You need to roll back your repository to HEAD~1
; the parent commits for the new commit from the remote repository. There are several ways of doing this.
You can use the git reset
command, as illustrated below.
$ git reset --hard HEAD~1
The git reset --hard
command will discard any uncommitted changes in your repository.
You can also use the SHA-1
of the parent commit. Let’s look at an example.
Here is the commit history for a repo after running the git pull
command.
$ git log --oneline
3b65c8e Update README.md
16b997a Update LICENSE.md
To move to the parent commit using the SHA-1
, we will run:
$ git reset --hard 16b997a
You can also reset to a certain point in time. If you run the git pull
twenty minutes ago, you can run the command below to restore the old version of your repository.
$ git reset --hard master@{"20 minutes ago"}
After pulling changes from the remote repository, you can revert your repository to the previous version using the git reset --hard
command. Stash any uncommitted work, as this command will also discard the changes.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn