How to Clean the Local Working Directory in Git
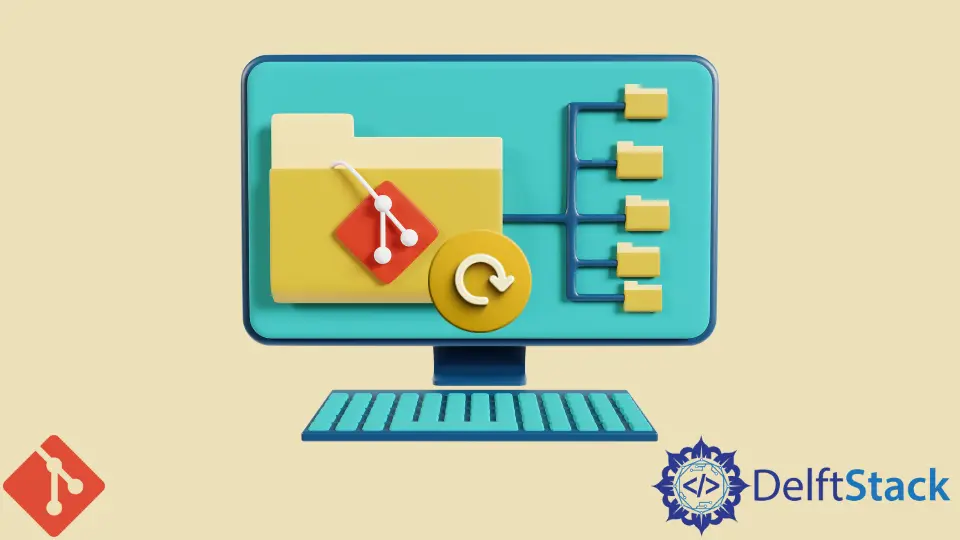
This article illustrates how you can clean your local working directory in Git. We will see how you can do away with uncommitted changes and untracked files.
We will also use the git clean
command to delete untracked folders. Let’s jump right in.
Clean the Local Working Directory in Git
Let’s take the working directory below as our working directory.
Let’s say we want to clean up everything on our working directory. That means discarding the changes made to the README.md
file and all the untracked files and folders.
How do we go about this?
There are several commands we can employ to deal with the modified file.
- We can use the
git checkout
command. - The
git restore <file>
command. - The
git reset --hard
command.
Check out the example below.
$ git checkout README.md
The command above will reset our README.md
file to the last committed state in our repository. It will do away with the uncommitted changes.
Example 2:
$ git restore README.md
Git suggests this command. It will discard the changes made to our README.md
file in our working directory.
Example 3:
$ git reset --hard
This command comes in handy when we have dozens of files in our working directory. Instead of checking out or restoring one by one, you can run the git reset --hard
command.
It will reset our repository to the last committed state.
We have seen how we can clean our staging area. What about the untracked files and folders?
Before deleting the untracked files, it is advisable to counter-check. You can run the git clean
command with the dry run option to see what files and folders will be discarded.
To do this, run:
$ git clean -n -d
Once you are sure you want to delete everything under untracked
, run the command below.
$ git clean -f
The command above will delete files. To delete the folders, run:
$ git clean -f -d
To do away with ignored files, run:
$ git clean -f -X
Here is an example.
In a nutshell, there are several commands we can use to clean our working directory in Git. The git clean
command deal with all untracked files and folders.
Always run a git clean
in dry run mode to check what will be deleted.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn