How to Commit and Push a Single File to the Remote in Git
- Understanding the Basics of Git
- Method 1: Using the Command Line Interface
- Method 2: Using a Git GUI Client
- Method 3: Using Python to Automate the Process
- Conclusion
- FAQ
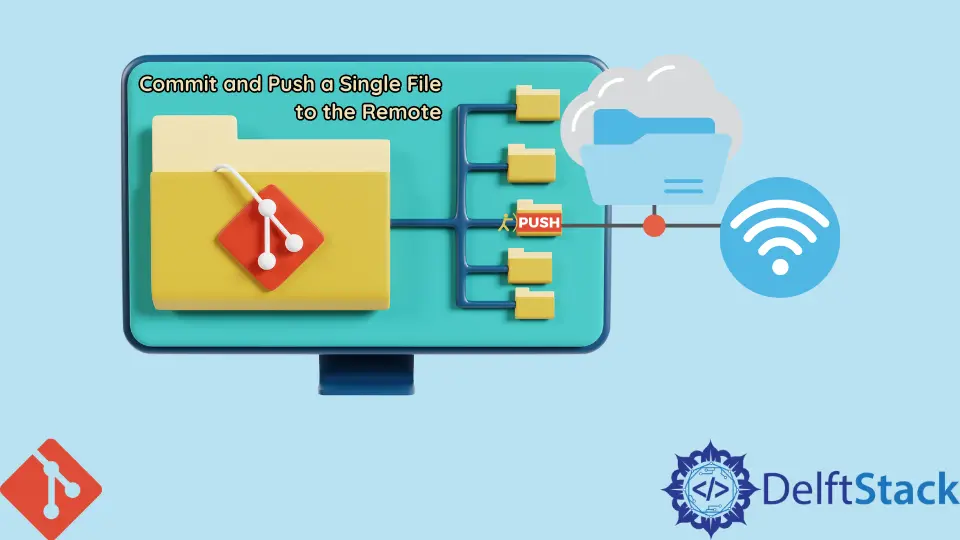
When working on a project with Git, you might find yourself in a situation where you only need to commit and push a single file to your remote repository. This can be especially useful when you’ve made a quick fix or added a new feature and want to keep your commits clean and focused.
In this article, we’ll walk you through the steps to commit and push a single file using Git, along with some handy Python code examples. By the end, you’ll not only understand how to do this efficiently but also why it’s a good practice to manage your commits in a structured way.
Understanding the Basics of Git
Before diving into the methods, let’s quickly recap what Git is and why it’s essential for version control. Git is a distributed version control system that allows multiple developers to work on a project simultaneously without interfering with each other’s work. It tracks changes in files and enables you to revert to previous versions if necessary.
Now, let’s explore how to commit and push a single file to your remote repository.
Method 1: Using the Command Line Interface
The command line is a powerful tool for managing your Git repositories. To commit and push a single file, you’ll need to follow a few simple steps.
First, navigate to your project directory in the terminal. Then, use the following commands:
git add path/to/your/file.py
git commit -m "Your commit message here"
git push origin branch-name
In this example, replace path/to/your/file.py
with the actual path to your file, and branch-name
with the name of the branch you want to push to.
Output:
[branch-name 1234567] Your commit message here
1 file changed, 10 insertions(+), 2 deletions(-)
The git add
command stages the specified file for commit, while git commit
creates a snapshot of your changes with a descriptive message. Finally, git push
uploads your commit to the remote repository, making your changes available to others.
Using the command line gives you precise control over what you’re committing and pushing, allowing for a more organized workflow. This method is particularly beneficial when you want to avoid pushing unnecessary changes or files.
Method 2: Using a Git GUI Client
If you prefer a graphical user interface over the command line, there are several Git GUI clients available that can simplify the process of committing and pushing files. Applications like SourceTree, GitKraken, or GitHub Desktop offer intuitive interfaces to manage your repositories.
Here’s how you can commit and push a single file using a Git GUI client:
- Open your Git GUI client and navigate to your repository.
- In the file list, locate the file you want to commit.
- Select the file and stage it for commit.
- Enter a commit message in the provided field.
- Click the commit button to save your changes.
- Finally, click the push button to upload your changes to the remote repository.
Output:
Commit successful. Pushed to origin/branch-name.
Using a GUI client can be especially helpful for those who are not as comfortable with the command line. It provides a visual representation of your repository, making it easier to understand the changes you’re making. Additionally, some clients offer features like merge conflict resolution and branch management, which can enhance your Git experience.
Method 3: Using Python to Automate the Process
If you often find yourself committing and pushing files, you might want to automate the process using Python. By leveraging the subprocess
module, you can create a script that performs these Git operations for you.
Here’s a simple example of how to do it:
import subprocess
def commit_and_push(file_path, commit_message, branch_name):
subprocess.run(['git', 'add', file_path])
subprocess.run(['git', 'commit', '-m', commit_message])
subprocess.run(['git', 'push', 'origin', branch_name])
commit_and_push('path/to/your/file.py', 'Your commit message here', 'branch-name')
Output:
[branch-name 1234567] Your commit message here
1 file changed, 10 insertions(+), 2 deletions(-)
In this script, the commit_and_push
function takes three parameters: the path to the file you want to commit, the commit message, and the branch name. The subprocess.run
function executes the Git commands as if you were typing them in the terminal.
This method is particularly useful for developers who prefer to integrate Git operations into their workflow seamlessly. You can even expand this script to include error handling or logging to make it more robust.
Conclusion
In summary, committing and pushing a single file to a remote repository in Git can be accomplished through various methods, including the command line, GUI clients, or even automation with Python. Each method has its advantages, and your choice may depend on your personal preferences or the specific requirements of your project. By mastering these techniques, you’ll enhance your Git workflow, ensuring that your commits are clean, organized, and easy to manage.
FAQ
-
How do I check the status of my Git repository?
You can check the status of your Git repository by running the commandgit status
in your terminal. This will show you which files are staged, unstaged, or untracked. -
Can I commit multiple files at once?
Yes, you can commit multiple files at once by adding them all in thegit add
command, like this:git add file1.py file2.py
. -
What happens if I forget to add a file before committing?
If you forget to add a file before committing, it won’t be included in that commit. You can always add the file and make a new commit afterward. -
Is it possible to undo a commit?
Yes, you can undo a commit using the commandgit reset HEAD~1
for the last commit orgit reset --soft <commit-hash>
for a specific commit. -
How can I view my commit history?
You can view your commit history by running the commandgit log
in your terminal. This will display a list of all commits in your repository.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn