How to Pull Specific Commit From Git Repository
- Understanding Git Commit IDs
- Pulling a Specific Commit Using Cherry-Pick
- Creating a New Branch from a Specific Commit
- Resetting to a Specific Commit
- Conclusion
- FAQ
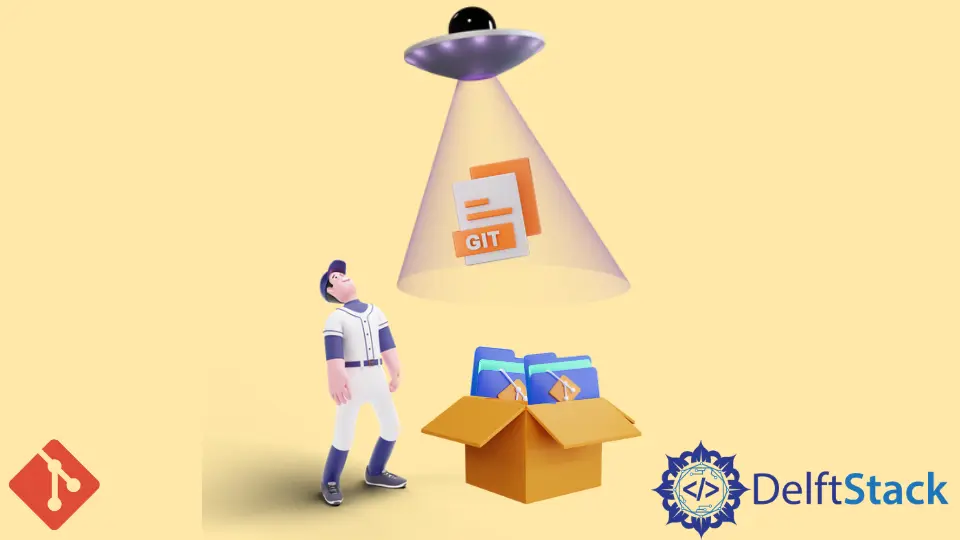
When working with Git, there are times when you may need to pull a specific commit from a remote repository. This can be particularly useful in collaborative projects where you want to revert to a previous state or integrate changes from a specific point in the project’s history.
In this article, we’ll explore various methods to pull a specific commit from a remote Git repository. Whether you’re a seasoned developer or just starting with Git, this guide will provide you with clear, actionable steps to achieve your goal efficiently.
Understanding Git Commit IDs
Before diving into the methods, it’s essential to understand what a Git commit ID is. Each commit in a Git repository has a unique identifier, known as a commit hash. This hash is a 40-character string that allows you to reference specific changes made in the repository. You can find the commit ID by running the command:
git log
This command displays a log of all commits, showing their hashes, authors, and commit messages. Once you have the commit ID you want to pull, you can proceed with the methods outlined below.
Pulling a Specific Commit Using Cherry-Pick
One of the most straightforward ways to pull a specific commit is by using the git cherry-pick
command. This command allows you to apply the changes introduced by a specific commit to your current branch. Here’s how you can do it:
git fetch origin
git cherry-pick <commit-id>
Replace <commit-id>
with the actual commit hash you want to pull. The git fetch origin
command updates your local repository with the latest changes from the remote repository without merging them. After fetching, git cherry-pick
applies the changes from the specified commit.
Output:
[main 1a2b3c4] Commit message of the cherry-picked commit
Using cherry-pick
is particularly beneficial when you want to integrate a single commit without merging an entire branch. However, be cautious, as conflicts may arise if the changes in the commit conflict with your current branch. In such cases, Git will prompt you to resolve the conflicts before you can complete the cherry-pick operation.
Creating a New Branch from a Specific Commit
If you prefer to create a new branch from a specific commit, you can do so with the following commands:
git fetch origin
git checkout -b new-branch-name <commit-id>
In this example, replace new-branch-name
with your desired branch name and <commit-id>
with the hash of the commit you want to pull. The git checkout -b
command creates a new branch and switches to it simultaneously.
Output:
Switched to a new branch 'new-branch-name'
This method is useful if you want to explore or work on changes from a specific commit without affecting the main branch. It allows you to isolate your work and make any necessary adjustments before merging back into the main branch later. However, remember that this new branch will only have the state of the repository as of the specified commit, so any subsequent commits on the original branch will not be included.
Resetting to a Specific Commit
If you want to reset your current branch to a specific commit, you can use the git reset
command. This method will remove all commits after the specified commit, effectively reverting your branch to that point in history. Use this command with caution, as it can lead to loss of work if not handled properly.
git fetch origin
git reset --hard <commit-id>
Replace <commit-id>
with the hash of the commit you want to reset to. The --hard
option means that your working directory will also be updated to match the specified commit.
Output:
HEAD is now at 1a2b3c4 Commit message of the reset commit
Using git reset
is a powerful way to undo changes, but it should be used with caution, especially in a collaborative environment. If you reset to a commit and then push to a shared branch, it can rewrite history, potentially causing issues for other collaborators. Always ensure that you communicate with your team before performing a hard reset.
Conclusion
Pulling a specific commit from a Git repository can be accomplished in several ways, depending on your needs. Whether you choose to cherry-pick a commit, create a new branch, or reset your current branch, understanding these methods will enhance your Git skills and improve your workflow. As you continue to work with Git, remember to always check the commit history and communicate with your team to ensure a smooth collaboration.
FAQ
-
How can I find the commit ID in Git?
You can find the commit ID by using the commandgit log
, which displays the commit history along with their hashes. -
What happens if I cherry-pick a commit with conflicts?
If there are conflicts when cherry-picking, Git will prompt you to resolve them before you can complete the operation. -
Can I pull multiple commits at once using cherry-pick?
Yes, you can cherry-pick multiple commits by specifying their commit IDs one after the other, or by using a range.
-
Is it safe to use git reset?
Usinggit reset
can be risky, especially with the--hard
option, as it can lead to loss of changes. Always ensure you have backups or communicate with your team. -
What is the difference between git reset and git revert?
git reset
changes the current branch to a specific commit, whilegit revert
creates a new commit that undoes the changes made by a previous commit.