How to Pull Latest Changes From Git Remote Repository to Local Branch
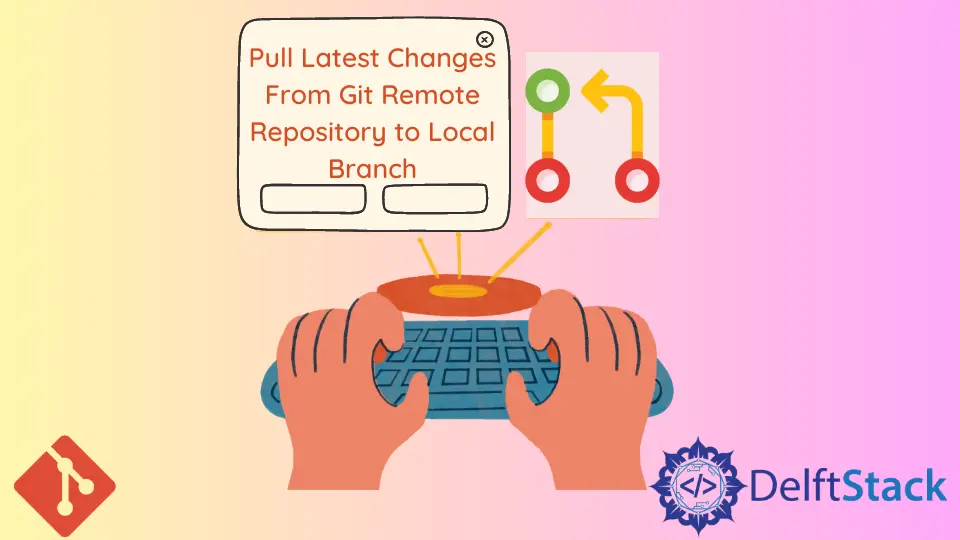
Suppose there is a branch named master
in the remote repository and another named development
in the local machine. Pulling all the changes the master branch has in the remote repository is pretty straightforward, but it might still bring issues in some cases.
Pull Latest Changes From Git Remote Repository to Local Branch
At first, you need to fetch all the information and changes that might be present in the remote repository. You can do that as follows:
git checkout master
It checkouts you from another branch to the master branch.
git fetch origin
The above commands pull the latest changes for us to see.
Fetch only downloads the new data, and it does not integrate any of the data included in your working files. However, it will give a fresh view of the things in the remote repository.
If there are changes, we can use the following command to ensure your local master repository is up-to-date with the remote master repository.
git pull origin master
After running the above command, you might run into merge conflict, which should be resolved, and the above command has to be rerun.
Now that you are up-to-date with the latest master branch, you can now check out to local development
branch as:
git checkout development
The HEAD is set to branch development
using this command, and now that you are on the desired branch where you want to pull the changes from the remote repository, you can run the following command to complete that.
git pull origin master
With this command, you have successfully merged the changes from the remote master
branch to the local development
branch. You might have to resolve merge conflicts, if any, though.